建立顺序表,实现初始化,删除,插入操作;
时间: 2024-09-19 22:06:53 浏览: 75
建立顺序表通常是在编程中创建一种线性数据结构,其中元素按照一定的顺序存储。以下是基本的操作步骤:
1. **初始化**:
- 定义一个顺序表,可以是一个数组或者动态数组(如C++的`std::vector`),需要指定初始容量。
```cpp
// C++
class SequentialList {
private:
int* elements; // 存储元素的指针
int size; // 当前元素的数量
int capacity; // 初始或最大容量
public:
SequentialList(int initialCapacity = 0) : capacity(initialCapacity) {
elements = new int[capacity];
size = 0;
}
```
2. **插入**:
- 如果还有空位,直接在列表末尾添加新元素。
```cpp
void insert(int value) {
if (size < capacity) {
elements[size++] = value;
} else {
// 扩容处理
resize(capacity * 2);
elements[size] = value;
size++;
}
}
void resize(int newSize) {
int* newElements = new int[newSize];
for (int i = 0; i < size; i++) {
newElements[i] = elements[i];
}
delete[] elements;
elements = newElements;
capacity = newSize;
}
```
3. **删除**:
- 删除指定位置的元素,需要注意更新索引和大小。
```cpp
void remove(int index) {
if (index >= 0 && index < size) {
for (int i = index; i < size - 1; i++) {
elements[i] = elements[i + 1];
}
size--;
if (size == capacity / 4 && capacity > 0) {
// 缩小数组以节省内存
resize(capacity / 2);
}
} else {
throw std::invalid_argument("Index out of range.");
}
}
```
阅读全文
相关推荐
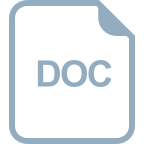
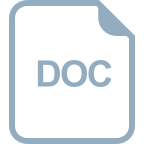
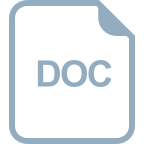















