unity 后台下载
时间: 2023-10-05 14:09:29 浏览: 286
在Unity中进行后台下载可以通过使用UnityWebRequest来实现。以下是一个简单的例子,演示了如何在后台下载文件:
```csharp
using UnityEngine;
using UnityEngine.Networking;
public class DownloadManager : MonoBehaviour
{
private UnityWebRequest webRequest;
private string downloadUrl = "https://www.example.com/file.zip";
private string savePath = "Assets/DownloadedFiles/file.zip";
public void StartDownload()
{
StartCoroutine(DownloadFile());
}
private IEnumerator DownloadFile()
{
webRequest = UnityWebRequest.Get(downloadUrl);
// 设置下载为后台模式
webRequest.SendWebRequest();
while (!webRequest.isDone)
{
// 可以在这里监听下载进度
float progress = webRequest.downloadProgress;
Debug.Log("Download progress: " + progress);
yield return null;
}
if (webRequest.result != UnityWebRequest.Result.Success)
{
// 下载失败,打印错误信息
Debug.LogError("Download failed: " + webRequest.error);
}
else
{
// 下载成功,保存文件到本地
System.IO.File.WriteAllBytes(savePath, webRequest.downloadHandler.data);
Debug.Log("Download completed!");
}
webRequest.Dispose();
}
}
```
在上面的例子中,`StartDownload` 方法会启动后台下载。`UnityWebRequest` 会在后台下载文件,并使用 `SendWebRequest` 方法开始下载。然后,在一个循环中,我们可以监听下载进度,当下载完成时,将文件保存到本地。
请注意,如果你希望在使用UnityWebRequest时在Android或iOS设备上进行后台下载,你需要确保在Unity的Player Settings中启用了"Run in Background"选项。
此外,还可以使用Asset Bundles或第三方插件来进行更高级的后台下载操作。但以上例子演示了一个基本的后台下载实现。
阅读全文
相关推荐
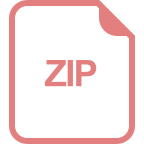






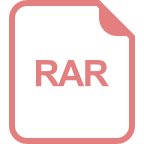
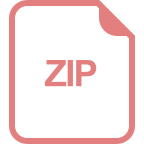
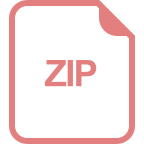
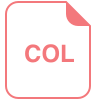



