typedef struct node { elemtype data; struct node* lchild, * rchild; }bstnode, * bst;用关键字序列插入的方法来创建二叉排序树,从终端或文件输入该序列。
时间: 2024-12-29 07:31:19 浏览: 7
在C语言中,`typedef`用来给已存在的数据类型起一个新的名字。这段代码首先定义了一个名为`bstnode`的结构体,表示二叉搜索树(BST)的节点,包含数据元素`data`、左孩子指针`lchild`和右孩子指针`rchild`。
然后,`bst`是一个别名,它代表`bstnode`类型的指针,可以用来指向BST的根节点。
下面是如何使用`typedef`和给定的结构体创建BST,并插入关键字序列的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义结构体和typedef
typedef struct node {
void* data;
struct node* lchild, * rchild;
} bstnode;
typedef bstnode* bst;
// 插入函数
bst insert(bst root, void* key) {
if (root == NULL) {
return (bst)malloc(sizeof(bstnode));
}
if (*((int*)key) < *((int*)root->data)) {
root->lchild = insert(root->lchild, key);
} else {
root->rchild = insert(root->rchild, key);
}
return root;
}
// 从输入获取并插入关键字序列
int main() {
FILE* file = fopen("input.txt", "r"); // 假设输入文件名是"input.txt"
if (file == NULL) {
printf("Error opening the file.\n");
return 1;
}
bst root = NULL;
while (!feof(file)) {
int key;
fscanf(file, "%d", &key);
root = insert(root, &key);
}
fclose(file); // 关闭文件
// 此处你可以添加对BST的操作,比如遍历、查找等
return 0;
}
```
这段代码首先打开文件,然后逐行读取整数并将它们作为关键字插入BST。注意,这里假设输入的数据都是整数,如果你的数据类型不同,需要相应地调整`fscanf`的格式字符串。
阅读全文
相关推荐
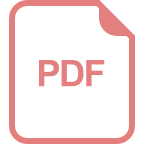
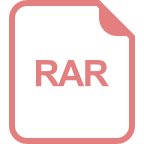
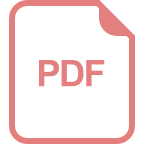
















