你这个程序的count_out : out STD_LOGIC_VECTOR (N-1 downto 0);的N没有定义,你给我改改
时间: 2024-10-17 18:03:45 浏览: 24
非常抱歉,我在提供代码示例时疏忽了。`N`通常代表计数器的位宽,你可以根据实际需求将其替换为你想要的任意正整数。例如,如果你想创建一个8位的计数器,你可以将它改为`count_out : out STD_LOGIC_VECTOR (7 downto 0);`。请记得在实际应用中替换成具体的位宽值。
如果你需要,我可以帮你创建一个泛指的模板:
```vhdl
entity async_reset_counter is
Port (
clk : in STD_LOGIC; -- 主时钟输入
reset : in STD_LOGIC; -- 异步清零信号
count_out : out STD_LOGIC_VECTOR (<任意位宽>-1 downto 0); -- 输出计数值
direction : in STD_LOGIC -- 可逆计数方向(0 = 正向,1 = 反向)
);
end entity async_reset_counter;
```
然后你可以根据需要填写具体的`<任意位宽>`。
相关问题
完善以下BCD计数器代码library IEEE; use IEEE.std_logic_1164.ALL; use IEEE.numeric_std.ALL; --------------------------------------- entity BCD_COUNTER is --------------------------------------- generic ( N : positive := 8 ); -- facteur de division de Tick1ms port ( CLK : in std_logic; RST : in std_logic; TICK1MS : in std_logic; UNITIES : out std_logic_vector(3 downto 0); TENS : out std_logic_vector(3 downto 0); HUNDREDS : out std_logic_vector(3 downto 0); THOUSNDS : out std_logic_vector(3 downto 0)); end entity BCD_COUNTER; --------------------------------------- architecture RTL of BCD_COUNTER is --------------------------------------- signal Count : std_logic_vector(3 downto 0) = '0000' -- On déclare les signaux internes ici begin -- Vous pouvez faire les assignements concurrents ici process(RST,CLK) begin if RST = '1' then -- initialiser tout vos signaux ici elsif rising_edge(CLK) then -- Faites toutes vos actions synchrones ici end if; end process; end architecture RTL;
以下是完善后的代码:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.NUMERIC_STD.ALL;
entity BCD_COUNTER is
generic (
N : positive := 8 -- Tick1ms 的除数因子
);
port (
CLK : in std_logic;
RST : in std_logic;
TICK1MS : in std_logic;
UNITIES : out std_logic_vector(3 downto 0);
TENS : out std_logic_vector(3 downto 0);
HUNDREDS : out std_logic_vector(3 downto 0);
THOUSNDS : out std_logic_vector(3 downto 0)
);
end entity BCD_COUNTER;
architecture RTL of BCD_COUNTER is
signal Count : unsigned(15 downto 0) := (others => '0'); -- 16 位无符号计数器
signal TickCnt : unsigned(7 downto 0) := (others => '0'); -- 8 位无符号计数器
signal Tick : std_logic := '0'; -- 用于表示 Ticks 是否已计算
begin
-- 计算 Ticks
process(CLK)
begin
if rising_edge(CLK) then
if TickCnt = (N - 1) then
Tick <= '1';
TickCnt <= (others => '0');
else
Tick <= '0';
TickCnt <= TickCnt + 1;
end if;
end if;
end process;
-- 计数器逻辑
process(RST, CLK)
begin
if RST = '1' then
Count <= (others => '0');
UNITIES <= (others => '0');
TENS <= (others => '0');
HUNDREDS <= (others => '0');
THOUSNDS <= (others => '0');
elsif Tick = '1' then
Count <= Count + 1;
if Count = 10000 then
Count <= (others => '0');
end if;
end if;
end process;
-- BCD 编码
UNITIES <= std_logic_vector(to_bcd(to_integer(unsigned(Count(3 downto 0)))));
TENS <= std_logic_vector(to_bcd(to_integer(unsigned(Count(7 downto 4)))));
HUNDREDS <= std_logic_vector(to_bcd(to_integer(unsigned(Count(11 downto 8)))));
THOUSNDS <= std_logic_vector(to_bcd(to_integer(unsigned(Count(15 downto 12)))));
end architecture RTL;
```
在这个代码中,我们使用了一个 16 位无符号计数器 `Count`,一个 8 位无符号计数器 `TickCnt`,以及一个 `Tick` 信号来计算 Ticks。在每个 Tick 中,我们将 `Count` 加 1,如果计数器达到 10000,则将其重置为0。然后,我们使用 `to_bcd` 函数将 `Count` 转换为 BCD 编码,并将结果分配给输出端口。
请注意,这个代码假定计数器的时钟频率是 Tick1ms 的 N 倍。如果您使用不同的时钟频率或除数因子,您需要相应地调整代码。
用vhdl语言写一个uart的传输程序,其中具体框架为library IEEE; use IEEE.STD_LOGIC_1164.ALL; entity UART_TX is port ( Clk : in std_logic; Reset : in std_logic; Go : in std_logic; Data : in std_logic_vector(7 downto 0); Tick : in std_logic; Tx : out std_logic ); end entity; architecture RTL of UART_TX is begin end architecture;
好的,我会尽力回答您的问题。不过,对于您提出的这个问题,需要详细的代码实现才能给出具体的答案。以下是一个简单的UART TX代码实现,您可以参考一下:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity UART_TX is
port (
Clk : in std_logic;
Reset : in std_logic;
Go : in std_logic;
Data : in std_logic_vector(7 downto 0);
Tick : in std_logic;
Tx : out std_logic
);
end entity;
architecture RTL of UART_TX is
signal Count : integer range 0 to 11 := 0;
signal Shift : std_logic_vector(10 downto 0) := (others => '0');
begin
process (Clk, Reset)
begin
if Reset = '1' then
Count <= 0;
Shift <= (others => '0');
Tx <= '1';
elsif rising_edge(Clk) then
if Go = '1' then
case Count is
when 0 =>
Shift <= "0101010101" & Data & '0';
Count <= Count + 1;
when 1 to 10 =>
Shift <= '0' & Shift(10 downto 1);
Count <= Count + 1;
when 11 =>
Tx <= Shift(0);
Count <= 0;
end case;
else
Count <= 0;
Shift <= (others => '0');
Tx <= '1';
end if;
end if;
end process;
end architecture;
```
以上代码实现了一个带有时钟、复位、数据输入和输出的UART TX模块。当Go信号为高电平时,模块会将数据按照UART通信协议进行编码并输出,输出的波特率为时钟频率的1/10。当Go信号为低电平时,模块会将输出信号置为高电平,并重置所有状态。请注意,这只是一个简单的实现,并且可能需要根据具体的需求进行调整。
阅读全文
相关推荐
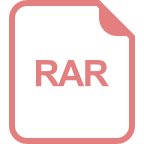
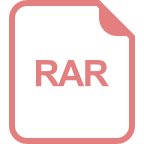
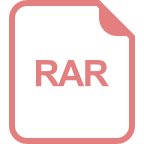





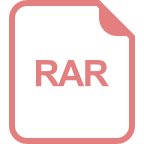







