写一段java程序,把hdfs的小文件合并成大文件
时间: 2024-10-14 09:02:40 浏览: 4
在Hadoop框架中,合并小文件通常会利用MapReduce API或者其他的工具如Apache Hadoop Streaming。下面是一个简单的Java MapReduce示例,用于将HDFS上的小文件合并成一个大的文本文件:
```java
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IOUtils;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class FileMerger {
public static void main(String[] args) throws IOException, InterruptedException {
if (args.length != 3) {
System.err.println("Usage: FileMerger <inputdir> <outputfile> <mapper>");
System.exit(2);
}
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Merge Small Files");
job.setJarByClass(FileMerger.class); // Set the JAR file for this job
job.setMapperClass(Mapper.class); // Define your Mapper class
job.setOutputKeyClass(Text.class); // Output key type is text
job.setOutputValueClass(Text.class); // Output value type is also text
// Input and output paths
FileInputFormat.addInputPath(job, new Path(args[0])); // The directory containing small files
FileOutputFormat.setOutputPath(job, new Path(args[1])); // The target output file
// Start the job
boolean success = job.waitForCompletion(true);
if (!success) {
System.err.println("Job failed!");
} else {
System.out.println("Job completed successfully.");
}
}
}
// 定义Mapper类,这里假设它从每个输入行读取并连接所有小文件内容
class Mapper extends Mapper<LongWritable, Text, NullWritable, Text> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
word.set(line);
context.write(null, word); // write the combined content to output
}
}
```
在这个例子中,`Mapper`类负责接收小文件的内容,然后将其写入到输出键值对中。记住,这只是一个基础示例,实际应用中可能需要处理更复杂的逻辑,比如拆分、排序和合并操作。
相关推荐
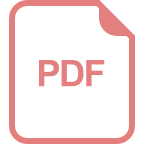
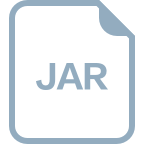
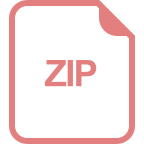















