vs2019中的yaml文件的读取和写入的c++
时间: 2024-09-24 20:05:34 浏览: 16
在Visual Studio 2019中使用C++处理YAML文件通常涉及到第三方库,比如`yaml-cpp`或者`ruamel.yaml`。这里我们以`yaml-cpp`为例说明如何读取和写入YAML文件。
首先,确保你已安装并包含了`yaml-cpp`库。你可以在项目的属性页(Project Properties -> C/C++ General -> Additional Include Directories)添加它的头文件路径。
### 1. 添加必要的库
```cpp
#include <yaml-cpp/yaml.h>
```
### 2. 读取YAML文件
```cpp
// 定义一个函数来读取文件
void readYaml(const std::string& filePath) {
YAML::Node node;
if (!yaml.load(filePath, node)) {
std::cerr << "Error loading YAML file: " << node.Mark.what() << std::endl;
return;
}
// 现在你可以遍历node访问数据
for (const auto& key : node.keys()) {
std::cout << key << ": " << node[key].as<std::string>() << std::endl;
}
}
int main() {
const std::string filePath = "path_to_your_yaml_file.yaml";
readYaml(filePath);
return 0;
}
```
### 3. 写入YAML文件
```cpp
// 定义一个函数来写入文件
void writeYaml(const YAML::Node& data, const std::string& filePath) {
bool success = yaml.dump(data, std::ofstream(filePath));
if (!success) {
std::cerr << "Error writing to YAML file." << std::endl;
}
}
// 在主函数中创建节点并写入
YAML::Node newData;
// ...填充数据
writeYaml(newData, "path_to_output.yaml");
```
相关推荐
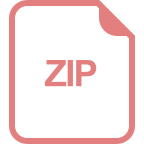
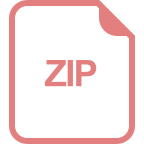
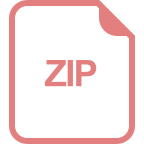














