string repalce c++
时间: 2023-10-28 19:58:04 浏览: 63
在C++中,你可以使用`std::string`类的成员函数`replace`来替换字符串中的子串。`replace`函数的原型为:
```cpp
std::string& replace(size_t pos, size_t count, const std::string& str);
```
其中,`pos`是要替换的子串的起始位置,`count`是要替换的子串的长度,`str`是用于替换的字符串。以下是一个示例:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::cout << "Original string: " << str << std::endl;
// 替换子串
str.replace(7, 5, "C++");
std::cout << "Replaced string: " << str << std::endl;
return 0;
}
```
输出结果为:
```
Original string: Hello, world!
Replaced string: Hello, C++!
```
在上面的示例中,我们将字符串中的子串"world"替换为"C++"。你可以根据自己的需求调整`pos`和`count`的值来实现不同的替换操作。
相关问题
c++string replace
C++ 中的 std::string 类有一个成员函数 replace(),可以用来替换字符串中的一些字符。
语法:
string.replace(position, length, string);
string.replace(iterator first, iterator last, string);
参数说明:
- position: 要替换的字符串的起始位置。
- length: 要替换的字符串的长度。
- string: 用来替换的字符串。
- iterator first: 要替换的字符串的起始位置(迭代器)
- iterator last: 要替换的字符串的终止位置(迭代器)
例子:
```
#include <iostream>
#include <string>
int main()
{
std::string str = "C++ is a programming language";
std::string toReplace = "programming";
std::string replaceWith = "powerful";
int pos = str.find(toReplace);
if (pos != std::string::npos)
{
str.replace(pos, toReplace.length(), replaceWith);
}
std::cout << str << std::endl;
return 0;
}
```
运行结果:
```
C++ is a powerful language
```
结论:
replace()函数可以在C++中的string类中替换一些字符。
c++ string replace
C++ 中的 std::string 类有一个成员函数 replace(),可以用来替换字符串中的一些字符。
语法:
string.replace(position, length, string);
string.replace(iterator first, iterator last, string);
参数说明:
- position: 要替换的字符串的起始位置。
- length: 要替换的字符串的长度。
- string: 用来替换的字符串。
- iterator first: 要替换的字符串的起始位置(迭代器)
- iterator last: 要替换的字符串的终止位置(迭代器)
例子:
```
#include <iostream>
#include <string>
int main()
{
std::string str = "C++ is a programming language";
std::string toReplace = "programming";
std::string replaceWith = "powerful";
int pos = str.find(toReplace);
if (pos != std::string::npos)
{
str.replace(pos, toReplace.length(), replaceWith);
}
std::cout << str << std::endl;
return 0;
}
```
运行结果:
```
C++ is a powerful language
```
结论:
replace()函数可以在C++中的string类中替换一些字符。
阅读全文
相关推荐
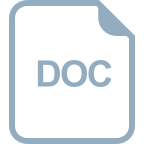
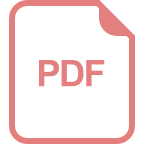
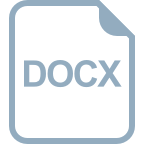













