float 数组线性插值代码
时间: 2024-10-21 07:02:50 浏览: 12
在Python中,你可以使用numpy库来进行float数组的线性插值。线性插值是一种简单的数值分析技术,它通过已知数据点计算出新数据点的估计值。下面是一个简单的例子:
```python
import numpy as np
# 假设我们有一个float数组x和对应的y值
x = np.array([0, 1, 2, 3])
y = np.array([0, 5, 10, 15])
# 定义一个新的插值点
new_point = 1.5
# 使用numpy的linspace函数创建插值范围
interp_range = np.linspace(x.min(), x.max(), num=100) # 创建均匀间隔的小数组
# 线性插值
interpolated_y = np.interp(interp_range, x, y)
# 计算并打印新的插值值
interpolated_value = interpolated_y[np.argmin(np.abs(new_point - interp_range))]
print(f"对于插值点 {new_point},线性插值的结果是:{interpolated_value}")
相关问题
如何用Fortran编写一个有注释的一维线性插值代码
以下是一个简单的Fortran程序,用于一维线性插值。该程序使用注释来说明其每个部分的功能。
```
program linear_interp
! This program demonstrates one-dimensional linear interpolation
implicit none
integer :: i, n
real :: x(10), y(10), x_interp, y_interp
! Define the data points
! In this example, we use 10 points with x ranging from 0 to 9 and y ranging from 0 to 90.
n = 10
do i = 1, n
x(i) = float(i-1)
y(i) = x(i) * 10.0
end do
! Enter the value to be interpolated
x_interp = 4.5
! Perform the linear interpolation
do i = 1, n-1
if (x_interp >= x(i) .and. x_interp <= x(i+1)) then
y_interp = y(i) + (y(i+1) - y(i)) * (x_interp - x(i)) / (x(i+1) - x(i))
exit
end if
end do
! Print the interpolated value
write(*,*) 'Interpolated value at x = ', x_interp, ' is ', y_interp
end program linear_interp
```
在这个程序中,我们首先定义了一个数组 `x` 和 `y`,它们表示我们要进行插值的数据点。然后,我们输入要插值的值 `x_interp`。接下来,我们使用一个循环来找到 `x_interp` 在 `x` 中对应的两个数据点,并使用这些数据点进行线性插值。最后,我们打印出插值结果。
在程序中,每个部分都有注释,这使得代码更易于理解和修改。
STM32 C语言 将80*62分辨率的数组 采用双线性插值到320*240 写出程序
要在STM32 C语言中使用双线性插值将80x62分辨率的图像数据转换到320x240分辨率,你需要创建一个函数来处理像素的插值计算,并遍历源图像逐行和每个目标像素点。这是一个简化的示例,假设我们有二维灰度图像数组`src_data[]`,源图像宽度和高度分别为80和62:
```c
#include <stdio.h>
#include <math.h>
// 双线性插值函数
void bilinear_interpolation(int src_data[][62], int x_target, int y_target, int src_width, int src_height, int dest_width, int dest_height) {
float u = (float)x_target / src_width;
float v = (float)y_target / src_height;
int x1 = floor(u);
int y1 = floor(v);
int x2 = x1 + 1;
int y2 = y1 + 1;
// 计算四个邻近像素的坐标
int src_x1 = x1 * src_width;
int src_y1 = y1 * src_height;
int src_x2 = src_x1 + src_width;
int src_y2 = src_y1 + src_height;
// 确保边界条件
if(x2 >= src_width || y2 >= src_height)
return;
float src1 = src_data[src_x1][src_y1];
float src2 = src_data[src_x1][src_y2];
float src3 = src_data[src_x2][src_y1];
float src4 = src_data[src_x2][src_y2];
float weight1 = (u - x1) * (v - y1);
float weight2 = (x2 - u) * (v - y1);
float weight3 = (u - x1) * (y2 - v);
float weight4 = (x2 - u) * (y2 - v);
int dest_index = y_target * dest_width + x_target;
dest_data[dest_index] = round(src1 * weight1 + src2 * weight2 + src3 * weight3 + src4 * weight4);
}
// 示例主函数,这里假设dest_data是一个已初始化的目标320x240像素数组
int main(void) {
int src_data[80][62]; // 源图像数据填充
for(y = 0; y < 240; ++y) {
for(x = 0; x < 320; ++x) {
bilinear_interpolation(src_data, x, y, 80, 62, 320, 240); // 插值并更新目标像素
}
}
return 0;
}
```
阅读全文
相关推荐
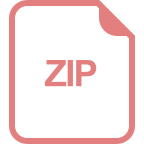
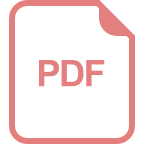
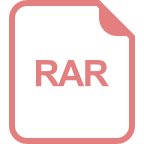
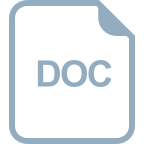
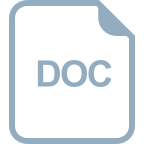
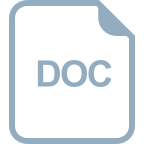
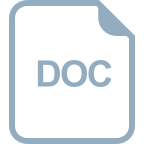
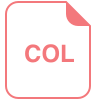
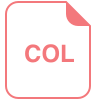
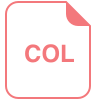







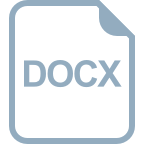