用C++ 设计一个算法,查找元素值为x的结点,设一棵二叉树以二叉链表作为它的存储表示,返回该结点地址及其父结点的地址
时间: 2024-12-14 11:24:19 浏览: 5
在C++中设计一个查找元素值为x的结点的算法,通常涉及深度优先搜索(DFS)或广度优先搜索(BFS),这里我会提供一种基于递归的深度优先搜索方案:
```cpp
#include <iostream>
using namespace std;
// 定义二叉链表节点结构
struct TreeNode {
int data;
TreeNode* left;
TreeNode* right;
TreeNode* parent; // 添加一个指向上一级节点的指针
};
// 用于递归查找函数
TreeNode* searchInBinaryTree(TreeNode* root, int x, TreeNode* parent = nullptr) {
if (root == nullptr)
return nullptr;
// 如果当前节点的数据就是目标值,返回当前节点地址和父节点地址
if (root->data == x)
return {root, parent};
// 递归查找左子树和右子树
TreeNode* leftResult = searchInBinaryTree(root->left, x, root);
TreeNode* rightResult = searchInBinaryTree(root->right, x, root);
// 如果在左右子树中找到结果,直接返回
if (leftResult || rightResult)
return leftResult ? leftResult : rightResult;
// 没有找到,说明x不在这个节点及其后代中
return nullptr;
}
int main() {
// 假设已经构建好了一个二叉链表
// ...
int targetValue = x; // 替换为你需要查找的目标值
TreeNode* result = searchInBinaryTree(root, targetValue);
if (result != nullptr) {
cout << "找到了节点,地址为: " << &result << ", 父节点地址为: " << result->parent << endl;
} else {
cout << "未找到节点" << endl;
}
return 0;
}
```
阅读全文
相关推荐
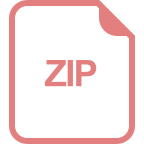
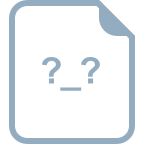
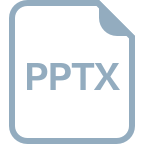















