torch.cat view
时间: 2023-11-18 19:53:10 浏览: 148
torch.cat 是 PyTorch 中的一个函数,用于将多个张量(tensor)拼接在一起。它的语法如下:
```python
torch.cat(tensors, dim=0, *, out=None) -> Tensor
```
其中,tensors 是要拼接的张量序列,dim 是指定拼接维度的参数,out 是输出张量(可选)。例如,我们有两个张量:
```python
import torch
x = torch.randn(2, 3)
y = torch.randn(2, 4)
```
我们可以将它们在第二个维度上拼接起来:
```python
z = torch.cat([x, y], dim=1)
print(z.shape) # 输出:torch.Size([2,7])
```
这里我们将 x 和 y 拼接在了第二个维度上,因此输出的张量 z 的形状是 [2, 7]。
相关问题
def decode_outputs(self, outputs, dtype): grids = [] strides = [] for (hsize, wsize), stride in zip(self.hw, self.strides): yv, xv = torch.meshgrid([torch.arange(hsize), torch.arange(wsize)]) grid = torch.stack((xv, yv), 2).view(1, -1, 2) grids.append(grid) shape = grid.shape[:2] strides.append(torch.full((*shape, 1), stride)) grids = torch.cat(grids, dim=1).type(dtype) strides = torch.cat(strides, dim=1).type(dtype) outputs[..., :2] = (outputs[..., :2] + grids) * strides outputs[..., 2:4] = torch.exp(outputs[..., 2:4]) * strides return outputs 在GPU环境进行速度优化 并提供代码示例
以下是一个使用CUDA并行计算的示例代码:
```python
import torch
class Decoder:
def __init__(self, hw, strides):
self.hw = hw
self.strides = strides
def decode_outputs(self, outputs, dtype):
grids = []
strides = []
for (hsize, wsize), stride in zip(self.hw, self.strides):
yv, xv = torch.meshgrid([torch.arange(hsize), torch.arange(wsize)])
grid = torch.stack((xv, yv), 2).view(1, -1, 2)
grids.append(grid)
shape = grid.shape[:2]
strides.append(torch.full((*shape, 1), stride))
grids = torch.cat(grids, dim=1).type(dtype).cuda()
strides = torch.cat(strides, dim=1).type(dtype).cuda()
outputs = outputs.cuda()
outputs[..., :2] = (outputs[..., :2] + grids) * strides
outputs[..., 2:4] = torch.exp(outputs[..., 2:4]) * strides
return outputs.cpu()
```
这段代码与之前的代码基本相同,只是在计算 grids 和 strides 时,将其转换为 CUDA 张量,并使用 GPU 进行计算。同时将模型输出 outputs 也转换为 CUDA 张量,并在计算后将其转换回 CPU 张量。这样可以在 GPU 环境下采用并行思维进行速度优化,提高代码的执行效率。
import torch x = torch.tensor([]) y = torch.tensor([1, 2, 3]) z = torch.cat((x, y), dim = 1)
这段代码会报错,因为在对 x 进行拼接时,x 是一个空的张量,没有指定维度。正确的写法应该是:
```
import torch
x = torch.tensor([])
y = torch.tensor([1, 2, 3])
z = torch.cat((x.view(1,-1), y.view(1,-1)), dim = 1)
```
这里使用了 `view` 方法将 x 和 y 的维度都转换成 `(1, -1)`,然后在第二维上进行拼接。
阅读全文
相关推荐
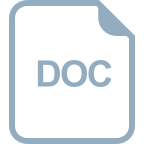
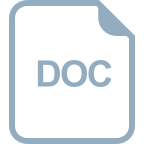
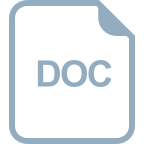













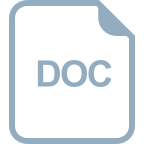