#include <pthread.h> #include <unistd.h>
时间: 2024-06-08 15:03:47 浏览: 269
`#include <pthread.h>` 和 `#include <unistd.h>` 是C语言中常用的预处理指令,它们分别包含了一些特定于线程和Unix-like系统(如Linux)的库函数和定义。
`#include <pthread.h>` 通常用于在C程序中创建和管理多线程。pthread库提供了一组API,允许开发者创建、启动、同步和终止线程。这些API提供了诸如线程优先级、线程同步(如互斥锁和条件变量)、线程属性等高级功能。
`#include <unistd.h>` 通常用于在C程序中实现Unix风格的进程间通信(IPC)。这个头文件包含了诸如 `sleep()`, `getpid()`, `fork()`, `execl()`, `wait()`, `pipe()`, `read()`, `write()` 等函数。这些函数允许程序在不同的进程之间进行通信,例如父子进程之间的通信,或者多个进程之间的同步。
简单来说,`#include <pthread.h>` 主要用于创建和管理多线程,而 `#include <unistd.h>` 主要用于实现进程间通信。这两者通常一起使用,以便在多线程程序中实现进程间的通信和同步。
相关问题
#include <pthread.h> #include <unistd.h>换成c++用的
`#include <pthread.h>` 和 `#include <unistd.h>` 是C和C++编程中常用的头文件,分别用于处理多线程和操作系统相关功能。不过在C++中,这两者头文件的功能可以被C++标准库中的一些头文件所替代。
对于`pthread.h`,它主要用于提供POSIX线程库(也被称为Pthreads),它提供了一种创建和管理线程的机制。在C++中,可以使用标准库中的 `std::thread` 类来替代这个库。`std::thread` 提供了一种更简单、更符合C++风格的线程创建和管理方式。
对于`unistd.h`,它包含了一些与Unix系统调用相关的函数,如 `fork`, `wait`, `read`, `write`, `close`, `fcntl`, `getpid`,等等。在C++中,这些功能通常可以通过标准库中的 `<cstdio>`, `<cstdlib>`, `<cerrno>` 等头文件中的函数来实现。
所以,如果你正在使用C++并且希望使用更现代、更符合C++风格的线程库,那么你应该将 `#include <pthread.h>` 替换为 `#include <thread>`,并且将 `<unistd.h>` 替换为 `<cstdlib>` 或 `<cstdio>` 等头文件。
请注意,这只是一种通用的建议,具体的替换方式可能会根据你的代码和项目需求有所不同。在某些情况下,可能需要使用特定的系统特定功能,这些功能可能在C++标准库中没有提供,那么你可能需要继续使用 pthread 或其他系统特定的库。
给以下代码写注释:#include <stdio.h> #include <stdlib.h> #include <pthread.h> #include <unistd.h> long int var = 0; void *tfn1(void *arg) { int i = var; for (int j = 0; j < 3; j++) { i = i + j; } printf("%d\n",i); pthread_exit((void *)i); return NULL; } int main(void) { pthread_t tid1; int *ret1; pthread_create(&tid1, NULL, tfn1, NULL); pthread_join(tid1, (void **)&ret1); printf("the result is %d\n",ret1); pthread_exit(NULL); }
注释如下:
```c
#include <stdio.h> // 标准输入输出库
#include <stdlib.h> // 标准库
#include <pthread.h> // POSIX线程库
#include <unistd.h> // 提供对 POSIX 操作系统 API 的访问
long int var = 0; // 声明一个全局变量 var,初值为 0
// 线程函数 tfn1,传入参数 arg,返回类型为 void*
void *tfn1(void *arg) {
int i = var; // 定义一个局部变量 i,初值为 var 的值
for (int j = 0; j < 3; j++) { // 循环 3 次,每次将 j 加到 i 上
i = i + j;
}
printf("%d\n",i); // 打印 i 的值
pthread_exit((void *)i); // 线程退出,并返回 i 的值
return NULL; // 返回 NULL
}
// 主函数
int main(void) {
pthread_t tid1; // 定义线程 tid1
int *ret1; // 定义返回值指针
pthread_create(&tid1, NULL, tfn1, NULL); // 创建线程,传入线程 id、属性、线程函数和参数
pthread_join(tid1, (void **)&ret1); // 等待线程结束,并获取线程返回值
printf("the result is %d\n",ret1); // 打印线程的返回值
pthread_exit(NULL); // 线程退出
}
```
阅读全文
相关推荐
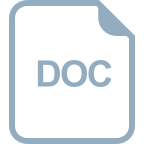
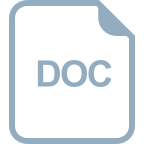
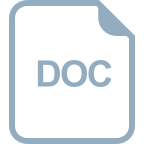














