头插法反转链表C语言代码
时间: 2024-04-21 12:19:53 浏览: 142
头插法是一种反转链表的方法,它通过将原链表的每个节点插入到新链表的头部,从而实现链表的反转。下面是使用头插法反转链表的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int val;
struct ListNode* next;
};
// 头插法反转链表
struct ListNode* reverseList(struct ListNode* head) {
struct ListNode* newHead = NULL; // 新链表的头节点
while (head != NULL) {
struct ListNode* nextNode = head->next; // 保存下一个节点的指针
head->next = newHead; // 将当前节点插入到新链表的头部
newHead = head; // 更新新链表的头节点
head = nextNode; // 移动到下一个节点
}
return newHead;
}
// 创建链表
struct ListNode* createList(int* nums, int size) {
struct ListNode* head = NULL;
struct ListNode* tail = NULL;
for (int i = 0; i < size; i++) {
struct ListNode* newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->val = nums[i];
newNode->next = NULL;
if (head == NULL) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
return head;
}
// 打印链表
void printList(struct ListNode* head) {
while (head != NULL) {
printf("%d ", head->val);
head = head->next;
}
printf("\n");
}
int main() {
int nums[] = {1, 2, 3, 4, 5};
int size = sizeof(nums) / sizeof(nums[0]);
struct ListNode* head = createList(nums, size);
printf("原链表:");
printList(head);
struct ListNode* newHead = reverseList(head);
printf("反转后的链表:");
printList(newHead);
return 0;
}
```
阅读全文
相关推荐

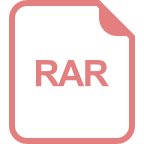
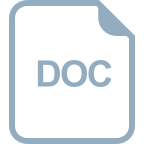
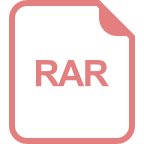
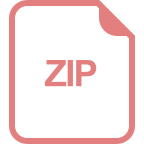
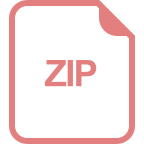
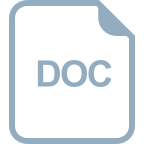
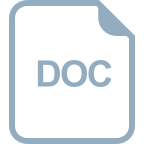
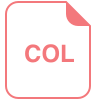
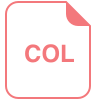
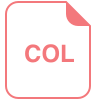
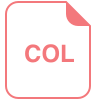



