请设计一个算法,判断该链表的全部n个字符是否中心对称,例如xyx,xyyx都是中心对称。c语言代码
时间: 2024-12-08 10:18:00 浏览: 24
为了判断一个链表的所有节点组成的字符串是否中心对称,我们可以将链表的一半存储到另一个新链表中,然后逐个比较两个链表的节点。如果它们对应位置的字符相同,说明字符串是对称的。以下是使用 C 语言实现的一个简单算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义链表节点结构体
typedef struct ListNode {
char data;
struct ListNode* next;
} ListNode;
// 创建链表的新节点
ListNode* createNode(char ch) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = ch;
newNode->next = NULL;
return newNode;
}
// 将链表复制到新链表
ListNode* copyList(ListNode* src, ListNode** dest) {
ListNode* head = *dest;
while (src) {
*dest = createNode(src->data);
(*dest)->next = head;
head = *dest;
src = src->next;
}
return head;
}
// 比较两个链表是否完全相同
bool compareLists(ListNode* list1, ListNode* list2) {
while (list1 && list2) {
if (list1->data != list2->data)
return false;
list1 = list1->next;
list2 = list2->next;
}
return !list1 && !list2;
}
// 判断链表是否中心对称
bool isCenterSymmetric(ListNode* head) {
ListNode* reverseCopyHead = copyList(head, &head); // 反转一半链表
return compareLists(head, reverseCopyHead);
}
int main() {
// 假设这里有一段链表
// 使用头插法创建链表,例如:head -> x -> y -> x -> y -> null
ListNode* head = createNode('x');
head->next = createNode('y');
head->next->next = createNode('x');
head->next->next->next = createNode('y');
if (isCenterSymmetric(head)) {
printf("链表是中心对称的。\n");
} else {
printf("链表不是中心对称的。\n");
}
return 0;
}
阅读全文
相关推荐









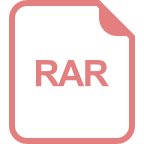
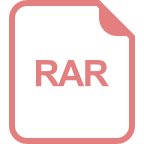

