哲学家进餐问题避免死锁解决方法Python
时间: 2023-12-14 21:33:09 浏览: 74
哲学家进餐问题是一个经典的同步问题,它描述了五个哲学家围坐在一张圆桌前,每个哲学家面前有一碗饭和一只筷子。哲学家的生活方式是交替地进行思考和进餐,当一个哲学家思考时,他不需要任何资源,但是当他想进餐时,他需要两只筷子。问题在于,如果每个哲学家都拿起自己左边的筷子,那么他们将永远无法进餐,因为每个人都在等待右边的筷子。这就是死锁。
解决哲学家进餐问题的方法有很多种,以下是其中的一些:
1. Chandy/Misra解法:这种方法使用了额外的“协调者”进程来协调哲学家们的进餐,从而避免了死锁。具体实现可以参考下面的Python代码:
```python
import threading
class Philosopher(threading.Thread):
def __init__(self, left_fork, right_fork):
threading.Thread.__init__(self)
self.left_fork = left_fork
self.right_fork = right_fork
def run(self):
while True:
self.left_fork.acquire()
locked = self.right_fork.acquire(False)
if locked:
break
self.left_fork.release()
else:
return
self.dine()
self.right_fork.release()
self.left_fork.release()
def dine(self):
print(f"{self.name} is eating")
forks = [threading.Lock() for n in range(5)]
philosophers = [Philosopher(forks[n], forks[(n + 1) % 5]) for n in range(5)]
for p in philosophers:
p.start()
```
2. 限制进餐人数:这种方法通过限制同时进餐的哲学家人数来避免死锁。具体实现可以参考下面的Python代码:
```python
import threading
class Philosopher(threading.Thread):
running = True
def __init__(self, left_fork, right_fork, count):
threading.Thread.__init__(self)
self.left_fork = left_fork
self.right_fork = right_fork
self.count = count
def run(self):
while self.running:
self.left_fork.acquire()
if not self.right_fork.acquire(False):
self.left_fork.release()
continue
self.dine()
self.right_fork.release()
self.left_fork.release()
def dine(self):
print(f"{self.name} is eating")
self.count -= 1
forks = [threading.Lock() for n in range(5)]
count = 2
philosophers = [Philosopher(forks[n], forks[(n + 1) % 5], count) for n in range(5)]
Philosopher.running = True
for p in philosophers:
p.start()
while True:
try:
input()
except KeyboardInterrupt:
break
Philosopher.running = False
for p in philosophers:
p.join()
```
3. 条件变量解法:这种方法使用了条件变量来协调哲学家们的进餐,从而避免了死锁。具体实现可以参考下面的Python代码:
```python
import threading
class Philosopher(threading.Thread):
def __init__(self, left_fork, right_fork, condition):
threading.Thread.__init__(self)
self.left_fork = left_fork
self.right_fork = right_fork
self.condition = condition
def run(self):
while True:
with self.condition:
self.condition.wait()
self.left_fork.acquire()
self.right_fork.acquire()
self.dine()
self.right_fork.release()
self.left_fork.release()
def dine(self):
print(f"{self.name} is eating")
forks = [threading.Lock() for n in range(5)]
condition = threading.Condition()
philosophers = [Philosopher(forks[n], forks[(n + 1) % 5], condition) for n in range(5)]
with condition:
condition.notifyAll()
for p in philosophers:
p.start()
while True:
try:
input()
except KeyboardInterrupt:
break
for p in philosophers:
p.join()
```
阅读全文
相关推荐
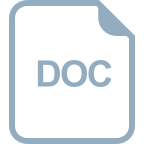




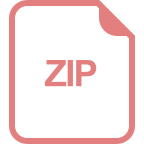
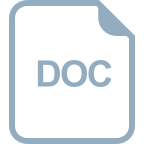








