哲学家进餐问题3种代码
时间: 2023-08-06 17:25:29 浏览: 113
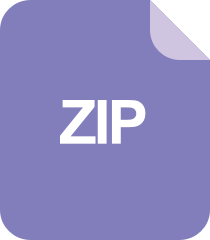
哲学家进餐问题的代码
好的,以下是哲学家进餐问题的三种代码实现:
1. 使用死锁避免机制的实现:
```python
import threading
class Chopstick:
def __init__(self):
self.lock = threading.Lock()
def pick_up(self):
self.lock.acquire()
def put_down(self):
self.lock.release()
class Philosopher(threading.Thread):
def __init__(self, left_chopstick, right_chopstick):
threading.Thread.__init__(self)
self.left_chopstick = left_chopstick
self.right_chopstick = right_chopstick
def run(self):
for i in range(1000):
self.left_chopstick.pick_up()
self.right_chopstick.pick_up()
# 吃饭
self.right_chopstick.put_down()
self.left_chopstick.put_down()
if __name__ == "__main__":
chopsticks = [Chopstick() for i in range(5)]
philosophers = [Philosopher(chopsticks[i], chopsticks[(i+1)%5]) for i in range(5)]
for philosopher in philosophers:
philosopher.start()
for philosopher in philosophers:
philosopher.join()
```
2. 使用超时机制的实现:
```python
import threading
class Chopstick:
def __init__(self):
self.lock = threading.Lock()
def pick_up(self):
self.lock.acquire()
def put_down(self):
self.lock.release()
class Philosopher(threading.Thread):
def __init__(self, left_chopstick, right_chopstick):
threading.Thread.__init__(self)
self.left_chopstick = left_chopstick
self.right_chopstick = right_chopstick
def run(self):
for i in range(1000):
self.pick_up_chopsticks()
# 吃饭
self.put_down_chopsticks()
def pick_up_chopsticks(self):
while True:
if self.left_chopstick.lock.acquire(timeout=1):
if self.right_chopstick.lock.acquire(timeout=1):
return True
else:
self.left_chopstick.lock.release()
else:
continue
def put_down_chopsticks(self):
self.left_chopstick.lock.release()
self.right_chopstick.lock.release()
if __name__ == "__main__":
chopsticks = [Chopstick() for i in range(5)]
philosophers = [Philosopher(chopsticks[i], chopsticks[(i+1)%5]) for i in range(5)]
for philosopher in philosophers:
philosopher.start()
for philosopher in philosophers:
philosopher.join()
```
3. 使用信号量的实现:
```python
import threading
class Chopstick:
def __init__(self):
self.lock = threading.Lock()
def pick_up(self):
self.lock.acquire()
def put_down(self):
self.lock.release()
class Philosopher(threading.Thread):
def __init__(self, left_chopstick, right_chopstick, semaphore):
threading.Thread.__init__(self)
self.left_chopstick = left_chopstick
self.right_chopstick = right_chopstick
self.semaphore = semaphore
def run(self):
for i in range(1000):
self.semaphore.acquire()
self.left_chopstick.pick_up()
self.right_chopstick.pick_up()
# 吃饭
self.right_chopstick.put_down()
self.left_chopstick.put_down()
self.semaphore.release()
if __name__ == "__main__":
chopsticks = [Chopstick() for i in range(5)]
semaphore = threading.Semaphore(4)
philosophers = [Philosopher(chopsticks[i], chopsticks[(i+1)%5], semaphore) for i in range(5)]
for philosopher in philosophers:
philosopher.start()
for philosopher in philosophers:
philosopher.join()
```
以上三种实现方式都可以解决哲学家进餐问题,其中第三种使用信号量的实现是最常用和最简洁的实现方式。
阅读全文
相关推荐
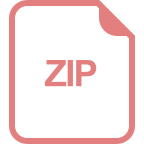















