用HC-SR04超声波测距模快STM32F103的代码
时间: 2024-09-14 09:07:05 浏览: 50
HC-SR04超声波传感器常用于测量物体的距离,而STM32F103是一款常见的微控制器,它支持GPIO口驱动超声波模块进行测距。以下是使用STM32F103控制HC-SR04的基本步骤和伪代码:
首先,需要配置STM32F103的GPIO口作为输入输出,以便发送和接收超声波信号。通常,TXD引脚负责触发脉冲,RXD引脚接收回音。
```c
// 定义GPIO初始化函数
void init_GPIO(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE); // 启动GPIOA时钟
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_7; // TXD
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; // 推挽输出
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6; // RXD
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_In_FLOATING; // 浮空输入
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
```
然后,设置发送和接收超声波脉冲的函数:
```c
// 发送超声波脉冲函数
void send_trigger(void) {
GPIO_SetBits(GPIOA, GPIO_Pin_7); // 高电平触发
HAL_Delay(10); // 等待10us
GPIO_ResetBits(GPIOA, GPIO_Pin_7); // 低电平结束
}
// 接收回音并计算距离
float measure_distance(void) {
uint16_t pulse_duration = 0;
GPIO_SetBits(GPIOA, GPIO_Pin_6); // 设置为输入模式
while (!(GPIO_ReadBit(GPIOA, GPIO_Pin_6))); // 等待开始标志
Timer_Start(); // 开始计时
while (GPIO_ReadBit(GPIOA, GPIO_Pin_6)); // 等待结束标志
Timer_Stop(); // 结束计时
pulse_duration = Timer_GetValue(); // 计算脉冲持续时间
float distance_cm = pulse_duration * 0.034 / 2; // 根据公式计算距离,假设声速343mm/us
return distance_cm;
}
```
最后,在主循环中定期调用`send_trigger`和`measure_distance`获取距离数据:
```c
int main(void) {
init_GPIO();
while (1) {
send_trigger();
float distance = measure_distance();
printf("Distance: %f cm\n", distance);
HAL_Delay(1000); // 每秒测量一次
}
}
```
注意,这只是一个基本框架,实际项目中还需要考虑错误处理、延时精度调整等因素,并且可能需要配合中断来提高响应速度。此外,这里的代码并未使用HAL库,如果你正在使用HAL库,那么GPIO操作和其他定时器操作会有所不同。
阅读全文
相关推荐
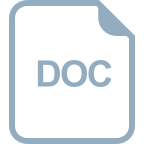
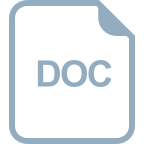
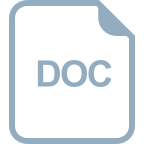

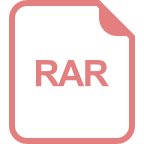
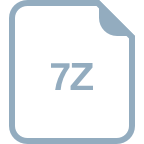
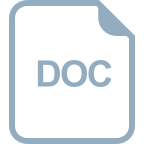

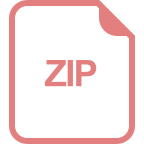
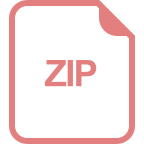
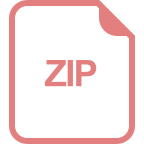
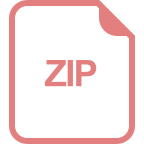
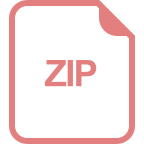
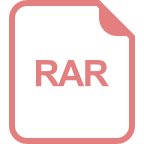
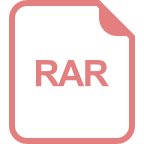



