c语言如何用read和write将文件拷贝到目标位置
时间: 2024-04-16 16:23:58 浏览: 20
要将一个文件的内容拷贝到另一个位置,你可以使用read和write函数结合循环来实现。下面是一个示例代码:
```c
#include <fcntl.h>
#include <unistd.h>
#define BUFFER_SIZE 4096
int main() {
int source_fd, dest_fd;
ssize_t bytesRead, bytesWritten;
char buffer[BUFFER_SIZE];
source_fd = open("source.txt", O_RDONLY); // 打开源文件,只读模式
if (source_fd == -1) {
perror("open source");
return 1;
}
dest_fd = open("destination.txt", O_WRONLY | O_CREAT | O_TRUNC, 0644); // 创建目标文件,写入模式
if (dest_fd == -1) {
perror("open destination");
close(source_fd);
return 1;
}
while ((bytesRead = read(source_fd, buffer, BUFFER_SIZE)) > 0) {
bytesWritten = write(dest_fd, buffer, bytesRead);
if (bytesWritten != bytesRead) {
perror("write");
close(source_fd);
close(dest_fd);
return 1;
}
}
if (bytesRead == -1) {
perror("read");
close(source_fd);
close(dest_fd);
return 1;
}
close(source_fd);
close(dest_fd);
return 0;
}
```
这个示例代码将打开一个名为source.txt的源文件和一个名为destination.txt的目标文件。然后,使用循环从源文件读取数据,并将数据写入目标文件,直到读取到文件末尾。注意要在open函数中使用合适的打开文件标志来创建目标文件,并确保写入的字节数与读取的字节数相等,以确保数据完整性。
请根据你的需求修改文件的名称和路径,并进行适当的错误处理。
相关推荐
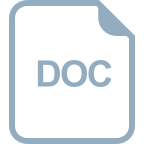














