cv2.findContours(thresh, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
时间: 2024-03-07 22:45:37 浏览: 26
cv2.findContours是OpenCV中的一个函数,用于在二值图像中查找轮廓。它的参数包括输入图像、轮廓检索模式和轮廓近似方法。
具体来说,cv2.findContours函数的参数如下:
- thresh:输入的二值图像,通常是通过阈值处理后得到的图像。
- cv2.RETR_LIST:轮廓检索模式,表示检测所有的轮廓,并将它们存储在列表中。
- cv2.CHAIN_APPROX_SIMPLE:轮廓近似方法,表示使用简单的轮廓近似方法来压缩轮廓信息。
该函数的返回值包括两个:轮廓列表和层次结构。轮廓列表是一个包含所有检测到的轮廓的数组,每个轮廓都是一个点集。层次结构是一个可选的输出参数,用于表示轮廓之间的关系。
相关问题
cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)参数
`cv2.findContours()`是OpenCV中用于查找图像轮廓的函数。它的参数包括:
- 第一个参数:二值化图像,即黑白图像,其中要查找轮廓的对象应该是白色,背景应该是黑色。
- 第二个参数:轮廓检索模式,有以下几种可选:
- `cv2.RETR_EXTERNAL`:只检测外轮廓。
- `cv2.RETR_LIST`:检测的轮廓不建立等级关系。
- `cv2.RETR_CCOMP`:检测轮廓并将其组织为两级层次结构。具体来说,顶层是物体的外部边界,第二层是物体内部的空洞边界,如果物体内部还有其他物体,则再次检测其外部边界并将其作为第三层,以此类推。
- `cv2.RETR_TREE`:检测轮廓并重建整个轮廓层次结构。
- 第三个参数:轮廓逼近方法,有以下几种可选:
- `cv2.CHAIN_APPROX_NONE`:存储所有的轮廓点,相邻的两个点之间的像素位置差不超过1。
- `cv2.CHAIN_APPROX_SIMPLE`:仅存储水平、垂直和对角线方向的端点,例如一个矩形轮廓只需要4个点来存储。
- `cv2.CHAIN_APPROX_TC89_L1`和`cv2.CHAIN_APPROX_TC89_KCOS`:使用Teh-Chinl链逼近算法中的一种。这些方法可以更好地逼近曲线,但计算成本更高。
下面是一个示例:
```python
import cv2
# 读取图像并转换为灰度图像
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化图像
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 查找轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 绘制轮廓
cv2.drawContours(img, contours, -1, (0, 0, 255), 3)
# 显示结果
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
contours, _ = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
This line of code finds the contours in a binary image.
The first argument is the binary image (thresh), which is the output of a thresholding operation.
The second argument is the contour retrieval mode, which specifies the hierarchy of contours to retrieve. cv2.RETR_TREE retrieves all of the contours and reconstructs a full hierarchy of nested contours.
The third argument is the contour approximation method, which simplifies the contour by removing redundant points. cv2.CHAIN_APPROX_SIMPLE compresses horizontal, vertical, and diagonal segments and leaves only their end points.
The function returns two values: a list of contours and the hierarchy of contours. The contours are represented as a list of points, where each point is a tuple of (x, y) coordinates. The hierarchy is represented as an array of four values: [Next, Previous, First Child, Parent].
相关推荐
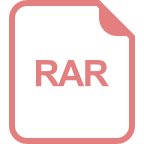












