编写爬虫,爬取学校官网新闻信息,并保存到本地,每个新闻保存一个文件。 网址 http://www.xpc.edu.cn/xwzx/xxxw1.htm
时间: 2024-11-07 19:29:50 浏览: 9
编写一个网站爬虫,用于抓取学校官网新闻并保存到本地,通常涉及以下几个步骤:
1. **安装必要的库**:首先,你需要安装Python的几个关键库,如`requests`用于发送HTTP请求获取网页内容,`BeautifulSoup`或`lxml`解析HTML文档,以及`os`和`json`用于文件操作。
```python
pip install requests beautifulsoup4
```
2. **分析目标页面结构**:打开`http://www.xpc.edu.cn/xwzx/xxxw1.htm`,了解新闻列表、单条新闻的URL以及所需数据的位置。这将帮助你编写对应的正则表达式或CSS选择器来提取信息。
3. **编写爬虫函数**:创建一个函数,接受URL作为输入,通过`requests.get()`获取页面源码,然后使用BeautifulSoup解析它。
```python
import requests
from bs4 import BeautifulSoup
def fetch_news(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 提取新闻链接和标题等信息
news_list = soup.select('.news-item') # 根据实际HTML结构修改选择器
for news in news_list:
title = news.find('h2', class_='title').text
link = news.find('a')['href']
save_to_file(title, link) # 调用另一个函数来保存新闻
def save_to_file(title, link):
filename = f'{title.replace(" ", "_")}.txt'
with open(filename, 'w', encoding='utf-8') as f:
f.write(f'Title: {title}\nLink: {link}\n')
```
4. **遍历获取更多页**:如果学校的新闻分页,需要循环访问下一页直到所有新闻都抓取完毕。你可以使用`find_all_next_page_link`这样的辅助函数找到并点击下一页链接。
5. **处理异常和错误**:确保捕获网络连接错误、解析错误等,并记录日志以便于调试。
6. **运行和清理**:最后,你可以通过调用`fetch_news`函数开始爬取,并在完成之后清理下载的文件。
```python
base_url = 'http://www.xpc.edu.cn/xwzx/'
start_url = base_url + 'xxxw1.htm'
# 获取所有新闻页并爬取
for i in range(1, total_pages+1): # 具体的total_pages需根据实际页面计算
fetch_news(start_url + str(i))
阅读全文
相关推荐
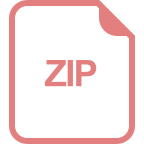
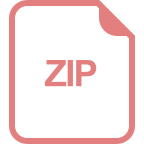
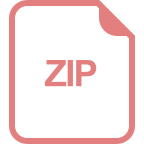

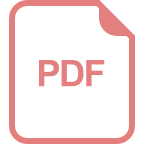
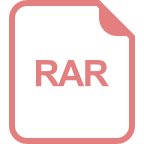
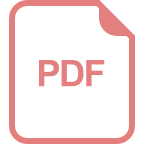
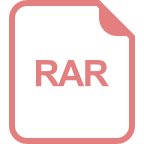
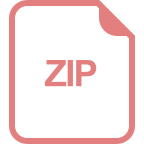
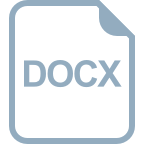
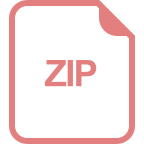
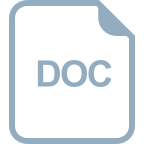
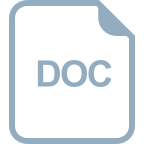
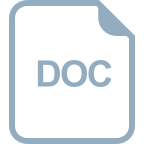
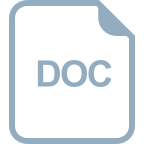


