利用c++实现编写有关二叉树的递归算法: (5)统计二叉树的宽度,即在二叉树的各层上具有结点数多的那一层上结点总数; (6)计算二叉树中各结点中的大元素的值; (7)交换每个结点的左孩子结点和右孩子结点; (8)从二叉树中删去所有叶子结点
时间: 2024-11-09 22:05:15 浏览: 45
在C++中,我们可以使用递归来处理二叉树的各种操作。以下是针对你提到的五个任务的示例代码:
1. **统计二叉树宽度** (假设`Node`是二叉树节点类,包含左右子节点指针):
```cpp
int maxWidth(Node* root) {
if (!root) return 0;
int leftWidth = getWidth(root->left);
int rightWidth = getWidth(root->right);
return std::max(leftWidth, rightWidth) + 1; // +1 for current node
}
int getWidth(Node* node) {
if (!node) return 0;
return maxWidth(node->left) > maxWidth(node->right) ? maxWidth(node->left) : maxWidth(node->right) + 1;
}
```
2. **查找最大值**:
```cpp
int findMaxValue(Node* root) {
if (!root) return INT_MIN;
return root->val > findMaxValue(root->left) && root->val > findMaxValue(root->right) ? root->val : std::max(findMaxValue(root->left), findMaxValue(root->right));
}
```
3. **交换节点的孩子**:
```cpp
void swapChildren(Node*& node) {
if (node && node->left && node->right) {
std::swap(node->left, node->right);
}
if (node->left) swapChildren(node->left);
if (node->right) swapChildren(node->right);
}
```
4. **删除所有叶子结点**:
```cpp
void removeLeaves(Node*& root) {
if (!root || !root->left && !root->right) { // 叶子节点
delete root;
root = nullptr;
} else {
removeLeaves(root->left);
removeLeaves(root->right);
}
}
```
注意,以上代码假设你已经实现了`Node`类,并且`val`表示节点的值。这些函数都是递归的,对于大型二叉树可能会消耗较多内存。
阅读全文
相关推荐
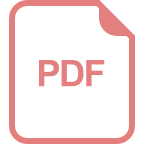
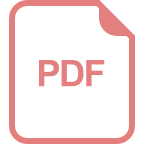
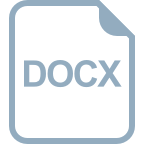



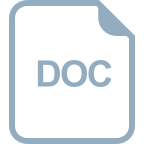
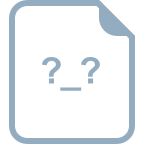
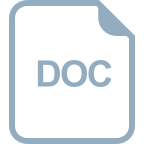






