添加定义延迟函数代码
时间: 2024-09-28 17:08:11 浏览: 28
在C语言中,没有直接提供“延迟函数”(类似JavaScript中的setTimeout)的功能,但你可以使用`<unistd.h>`库里的`sleep()`函数来模拟这种效果。`sleep(n)`会让程序暂停执行指定的时间(单位为秒),达到延迟的效果。
如果你想在某个时间点执行函数,而不是立即执行,一种常见的做法是在主函数中安排一个定时器,或者在一个单独的线程里运行这个函数,等待特定时间后再唤醒或调用它。这里有一个简单的例子,展示了如何在一个线程中延迟后执行某个函数:
```c
#include <stdio.h>
#include <pthread.h>
// 延迟后的函数原型
void delayed_function(int seconds);
// 主要的延时函数
void *delay_thread(void *arg) {
int seconds = (int)arg;
sleep(seconds); // 暂停指定秒数
printf("Delay over, calling 'delayed_function'\n");
delayed_function(seconds);
return NULL;
}
// 待延迟执行的函数
void delayed_function(int seconds) {
printf("Delayed function ran after %d seconds.\n", seconds);
}
int main() {
pthread_t delay_thread_id; // 创建一个新的线程
// 启动延时线程,传递延迟时间作为参数
if (pthread_create(&delay_thread_id, NULL, delay_thread, (void*)5)) { // 假设延迟5秒
perror("Error creating thread");
exit(1);
}
// 这里主线程继续执行,而延时函数将在指定时间后启动
printf("Main thread is running...\n");
// 等待线程完成
pthread_join(delay_thread_id, NULL);
return 0;
}
```
在这个例子中,`main`函数创建了一个新的线程,该线程会在5秒钟后调用`delayed_function`。注意,你需要包含必要的头文件并链接到支持线程的库,比如`-lpthread`。
阅读全文
相关推荐
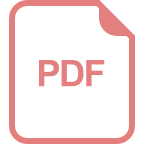
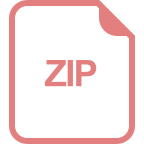
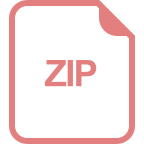




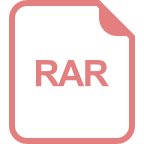
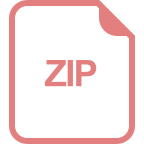
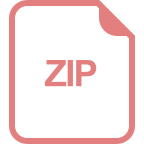
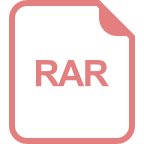
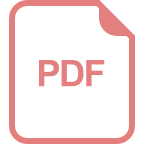
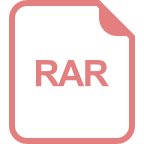
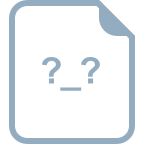
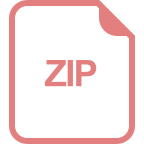



