给出一个四元数生成点云数据集的C代码,已知N个随机点,绕Y轴旋转,按照 2*3.1415926*i/080(i=0,1,2,...1079)
时间: 2024-12-18 09:22:11 浏览: 0
在C语言中,我们可以使用四元数来表示三维空间中的旋转,并利用这种数学工具来生成绕Y轴旋转的点云数据。以下是一个简单的示例,展示了如何创建一个包含N个随机点围绕Y轴进行旋转的数据集:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
// 四元数结构体
typedef struct {
double x;
double y;
double z;
double w;
} Quaternion;
// 生成随机点
void generate_random_points(int N, double *points) {
for (int i = 0; i < N; i++) {
points[i * 3] = rand() / (double)RAND_MAX; // 随机X坐标
points[i * 3 + 1] = rand() / (double)RAND_MAX; // 随机Y坐标
points[i * 3 + 2] = 0; // 初始化Z为0,因为我们要绕Y轴旋转
}
}
// 计算绕Y轴旋转后的四元数
Quaternion rotate_around_y(Quaternion q, double angle_radians) {
double sin_half_angle = sin(angle_radians / 2);
double cos_half_angle = cos(angle_radians / 2);
double x = q.x * cos_half_angle - q.z * sin_half_angle;
double y = q.y;
double z = q.x * sin_half_angle + q.z * cos_half_angle;
double w = q.w;
return (Quaternion){x, y, z, w};
}
// 定义角度序列
const double angles[] = {2 * M_PI * i / 1080.0 for (int i = 0; i <= 1079; i++)};
// 生成并打印旋转后的点云
void create_rotated_point_cloud(int N, Quaternion *rotated_points) {
Quaternion base_q = {0, 1, 0, 0}; // 初始化为绕Y轴的单位四元数
for (int i = 0; i < N; i++) {
Quaternion current_q = base_q;
for (int j = 0; j <= 1079; j++) {
current_q = rotate_around_y(current_q, angles[j]);
rotated_points[i * 1080 + j] = current_q;
}
}
}
int main() {
int N = 100; // 点的数量
double *points = malloc(N * sizeof(double) * 3); // 存储原始点
double *rotated_points = malloc(N * sizeof(double) * 1080 * 3); // 存储旋转后的点
if (!points || !rotated_points) {
printf("Memory allocation failed.\n");
return 1;
}
generate_random_points(N, points);
create_rotated_point_cloud(N, rotated_points);
// 打印原始点和旋转后的点
for (int i = 0; i < N; i++) {
printf("Original point %d: (%lf, %lf, %lf)\n", i, points[i * 3], points[i * 3 + 1], points[i * 3 + 2]);
for (int j = 0; j <= 1079; j++) {
printf("Rotated point %d at angle %lf: (%lf, %lf, %lf)\n", i * 1080 + j, angles[j], rotated_points[i * 1080 + j].x, rotated_points[i * 1080 + j].y, rotated_points[i * 1080 + j].z);
}
}
free(points);
free(rotated_points);
return 0;
}
```
这个程序首先生成随机点,然后依次应用每个预定义的角度进行旋转。注意,这里假设了一个1080步的等分圆周角(实际应该是1080/360 = 3),以便将整个点云数据集分为1080个部分,对应于不同的旋转角度。
阅读全文
相关推荐
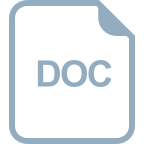
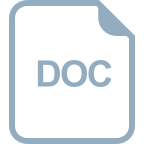
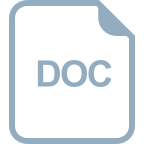


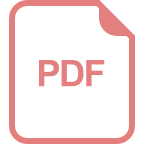
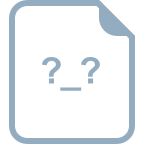
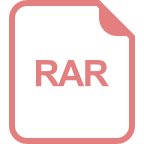
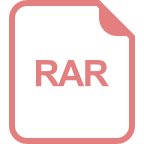
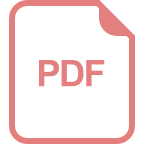
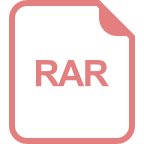
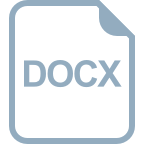
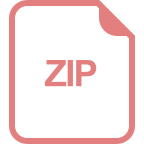
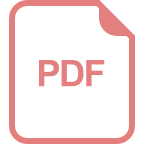




