BigInteger hex1 = new BigInteger("0x1F") 会报错at java.lang.NumberFormatException
时间: 2024-10-28 18:09:20 浏览: 21
创建 `BigInteger` 对象时,传入的字符串需要是有效的十六进制形式。如果遇到 `NumberFormatException`,通常是因为提供的字符串不符合十六进制的语法或者超出 `BigInteger` 的范围。`0x1F` 在十六进制中是正常的一个值,但在某些情况下可能会引发错误:
- **无效的字符**:如果你传入的字符串包含非十六进制字符,比如字母不是"A-F"或"a-f",或者数字超过0-9,那么就会抛出异常。
- **超出范围**:虽然 `BigInteger` 可以表示非常大的整数,但如果十六进制数值过大以至于无法精确地用 `BigInteger` 表示,也可能导致此异常。
确保字符串仅包含有效的十六进制字符,并且大小不超过 `BigInteger` 的最大容量,一般来说就不会有问题。如果仍然报错,检查一下输入的十六进制字符串是否正确。
```java
try {
BigInteger hex1 = new BigInteger("0x1F", 16);
} catch (NumberFormatException e) {
System.out.println("Invalid hexadecimal format or value too large.");
}
```
这里第二个参数16指定了字符串的基数是16,告诉 `BigInteger` 这是一个十六进制数。
相关问题
SQL 错误 [S1009]: java.lang.ClassCastException: java.math.BigInteger cannot be cast to java.lang.Long java.lang.ClassCastException: java.math.BigInteger cannot be cast to java.lang.Long java.math.BigInteger cannot be cast to java.lang.Long
这是一个 SQL 错误,具体原因是因为 java.math.BigInteger 类型无法强制转换为 java.lang.Long 类型。可能是在 SQL 查询中使用了错误的数据类型或者数据类型不匹配导致的。需要检查 SQL 查询语句和数据类型是否正确。
// Press Shift twice to open the Search Everywhere dialog and type `show whitespaces`, // then press Enter. You can now see whitespace characters in your code. import java.math.BigInteger; public class Main { public static void main(String[] args) { // Press Alt+Enter with your caret at the highlighted text to see how // IntelliJ IDEA suggests fixing it. BigInteger num1 = new BigInteger("5160000000000000000"); BigInteger num2= new BigInteger("4733944950000000000"); BigInteger sum = (num1 / num2) -1; } }
这段代码有一个错误。BigInteger类不支持使用算术运算符进行计算,需要使用它提供的方法来实现。在这里,我们需要使用BigInteger的divide方法来计算两个大整数的商。
以下是修改后的代码:
```
import java.math.BigInteger;
public class Main {
public static void main(String[] args) {
BigInteger num1 = new BigInteger("5160000000000000000");
BigInteger num2 = new BigInteger("4733944950000000000");
BigInteger quotient = num1.divide(num2);
BigInteger sum = quotient.subtract(BigInteger.ONE);
System.out.println(sum);
}
}
```
在这个例子中,我们首先使用BigInteger类创建两个大整数num1和num2。然后,我们使用divide方法计算num1除以num2的商,并将结果存储在BigInteger对象quotient中。最后,我们使用subtract方法计算quotient减去1的值,并将结果存储在BigInteger对象sum中。最后,我们将结果打印到控制台上。
阅读全文
相关推荐
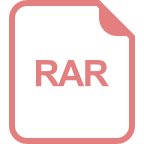
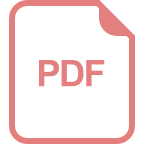
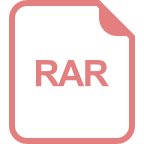




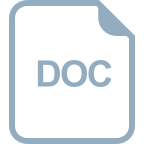








