L.tileLayer(tileUrl.Vect.TIANDITU,{subdomains:["t0","t1","t2","t3","t4","t5","t6","t7"]}),
时间: 2024-06-04 17:05:29 浏览: 6
L.tileLayer是Leaflet中用于加载瓦片地图的函数。其中tileUrl参数是一个字符串类型,代表了瓦片图层的URL地址,用于指定需要加载的地图服务。而tileUrl.Vect.TIANDITU则是指天地图矢量地图服务的URL地址。subdomains参数是一个数组类型,用于指定服务器的子域名。在请求瓦片时,Leaflet会轮流使用这些子域名来进行负载均衡,以减轻服务器的压力,提高用户体验。
相关问题
单片机 串口 波特率 c语言 9600 11.0592mhz t1,PLC与单片机串口通信的实现
要实现PLC与单片机串口通信,需要注意以下几个方面:
1. 波特率的设置:PLC和单片机之间的串口通信需要保证波特率相同,常用的波特率有9600、19200等,根据实际需求进行选择。在单片机中,可以通过设置波特率寄存器来设置波特率,如下所示:
```c
// 设置波特率为9600
UBRRH = 0;
UBRRL = 71;
```
其中,UBRRH和UBRRL是两个8位寄存器,用来设置波特率。上面的代码将波特率设置为9600,时钟频率为11.0592MHz,可以根据实际情况进行修改。
2. 数据格式的设置:PLC和单片机之间的数据格式需要保持一致,包括数据位、停止位、校验位等。常用的数据格式是8N1,即8个数据位、无校验位、1个停止位。在单片机中,可以通过设置UCSRC寄存器来设置数据格式,如下所示:
```c
// 设置数据格式为8N1
UCSRC = (1 << URSEL) | (1 << UCSZ1) | (1 << UCSZ0);
```
其中,URSEL是用来选择UCSRC寄存器的位,UCSZ1和UCSZ0分别表示数据位数,可以根据实际需求进行修改。
3. 串口中断的设置:在单片机中,可以通过设置USART_RXCIE寄存器来使能串口接收中断。当有数据从串口输入时,会触发中断,用户可以在中断服务函数中读取数据并进行处理。例如:
```c
// 使能串口接收中断
UCSRB |= (1 << RXCIE);
// 串口接收中断服务函数
ISR(USART_RXC_vect)
{
char data = UDR;
// 处理接收到的数据
}
```
4. 数据的发送和接收:在单片机中,可以通过UDR寄存器来进行数据的发送和接收。例如,要发送一个字符,可以将字符写入UDR寄存器:
```c
// 发送一个字符
UDR = 'A';
```
要接收一个字符,可以从UDR寄存器中读取数据:
```c
// 接收一个字符
char data = UDR;
```
通过以上步骤,就可以实现PLC与单片机之间的串口通信了。用户可以根据实际需求进行修改和扩展。
from sklearn.feature_extraction import DictVectorizer vect = DictVectorizer() features = features.to_dict(orient = 'records')
这段代码的作用是将一个 Pandas DataFrame 中的特征转换为字典格式,然后利用 DictVectorizer 进行向量化处理。具体来说:
- `features.to_dict(orient='records')` 将 DataFrame 中的每行特征转换为一个字典,其中键为特征名,值为对应的特征值。orient='records' 表示每行特征转换为一个字典,返回一个列表,每个元素为一行特征对应的字典。
- `vect = DictVectorizer()` 创建一个 DictVectorizer 对象。
- `features = vect.fit_transform(features)` 调用 DictVectorizer 的 `fit_transform()` 方法,将特征字典列表转换为一个稀疏矩阵,每行代表一个样本,每列代表一个特征。矩阵中的元素表示该样本在对应特征上的取值。
- 最终返回稀疏矩阵 `features`。这个矩阵可以作为输入给机器学习模型进行训练或预测。
相关推荐
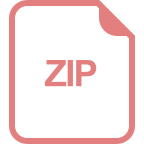
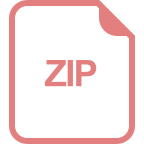












