python头歌英文词频统计
时间: 2024-12-17 18:22:41 浏览: 1
在Python中,可以使用`collections`模块下的`Counter`类来统计英文歌词中的单词频率。`Counter`是一个字典子类,用于计数可哈希对象(如字符串)。以下是简单的步骤:
```python
from collections import Counter
# 假设歌词存储在一个字符串list或单个字符串变量lyrics中
lyrics = "your song lyrics here"
# 将歌词分割成单词列表
word_list = lyrics.lower().split()
# 使用Counter统计每个单词的频率
word_freq = Counter(word_list)
# 输出最常见的前几个单词及其频率
most_common_words = word_freq.most_common(5)
print("最常见的单词及频率:", most_common_words)
```
在这个例子中,我们首先将所有歌词转换为小写并按空格分割成单词列表。然后使用`Counter`计算每个单词的出现次数。`most_common(n)`函数会返回出现次数最多的n个元素及其频率。
相关问题
用Python实现英文文本词频统计
可以使用Python的`collections`模块来实现英文文本的词频统计。下面是一个简单的示例代码:
```python
import re
from collections import Counter
def word_frequency(text):
# 使用正则表达式将文本中的非字母字符替换为空格
text = re.sub(r'[^a-zA-Z]', ' ', text)
# 将文本转换为小写,并按空格分割成单词列表
words = text.lower().split()
# 使用Counter对象统计单词出现的次数
word_counts = Counter(words)
return word_counts
# 示例用法
text = "Hello, how are you? I'm doing great, thank you!"
frequency = word_frequency(text)
print(frequency)
```
运行以上代码,输出结果为:
```
Counter({'hello': 1, 'how': 1, 'are': 1, 'you': 1, "i'm": 1, 'doing': 1, 'great': 1, 'thank': 1, 'you': 1})
```
以上代码首先使用正则表达式将非字母字符替换为空格,然后将文本转换为小写,并按空格分割成单词列表。接着使用`Counter`对象统计单词出现的次数,并返回结果。
python英文歌曲中的词频统计
在Python中,你可以使用一些文本处理库如NLTK(Natural Language Toolkit)和collections模块来进行英文歌曲歌词的词频统计。以下是一个简单的步骤:
1. **下载和导入必要的库**:
```python
import nltk
from collections import Counter
```
2. **读取歌词文件**:
如果歌词存储在一个.txt或其他文本文件里,可以用`open()`函数读取内容。
3. **预处理文本**:
- 分词:使用nltk的`word_tokenize()`方法将歌词分割成单词列表。
- 去除停用词和标点符号:可以使用`nltk.corpus.stopwords`中的停用词列表,并使用`string.punctuation`去除标点。
```python
nltk.download('punkt')
from nltk.corpus import stopwords
stop_words = set(stopwords.words('english'))
lyrics = open('song_lyrics.txt', 'r').read().lower()
words = [word for word in nltk.word_tokenize(lyrics) if word.isalpha() and word not in stop_words]
```
4. **词频统计**:
使用`Counter`计算每个单词出现的次数。
```python
word_freq = Counter(words)
most_common_words = word_freq.most_common()
```
5. **输出结果**:
可以打印出最常见的前几个单词及其频率,或者将结果保存到CSV或JSON文件中。
```python
for word, freq in most_common_words[:10]:
print(f"{word}: {freq}")
```
阅读全文
相关推荐
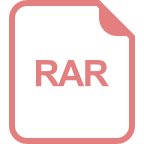
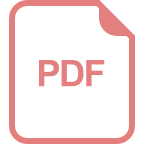
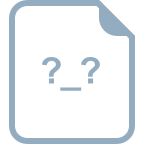
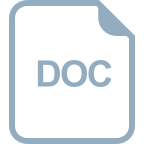
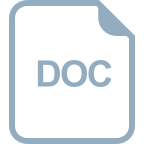


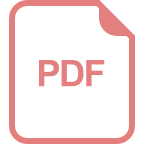
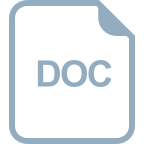







