编写纯c++代码,要求只使用cmath头文件,已知高度,倾角,航向,合速度,拟合一个抛物线,输入时间t,根据公式输出t时刻物体的三维坐标信息
时间: 2024-12-29 12:36:36 浏览: 41
为了编写一个纯C++代码来模拟物体在重力影响下的抛物线运动,我们需要首先了解几个关键点。物体的三维坐标由三个分量组成:水平(x),垂直(y)和沿着航向的方向(z)。考虑到航向角,我们可以将运动分解成水平、垂直和航向三个维度。以下是一个简单的代码示例,它只使用`cmath`库来进行三角函数和浮点数运算:
```cpp
#include <iostream>
#include <cmath>
// 定义常量
const double g = 9.8; // 重力加速度 (m/s²)
// 函数声明
void calculate_position(double height, double launch_angle, double heading_angle, double initial_speed, double* x, double* y, double* z, double time);
int main() {
double height, launch_angle, heading_angle, initial_speed, t;
std::cout << "请输入物体的高度 (m): ";
std::cin >> height;
std::cout << "请输入发射角度 (弧度): ";
std::cin >> launch_angle;
std::cout << "请输入航向角 (弧度): ";
std::cin >> heading_angle;
std::cout << "请输入初始速度 (m/s): ";
std::cin >> initial_speed;
std::cout << "请输入时间 (s): ";
std::cin >> t;
double x, y, z;
calculate_position(height, launch_angle, heading_angle, initial_speed, &x, &y, &z, t);
std::cout << "在时间 " << t << " 秒时,三维坐标为: (" << x << ", " << y << ", " << z << ")\n";
return 0;
}
// 计算每个维度的坐标
void calculate_position(double height, double launch_angle, double heading_angle, double initial_speed, double* x, double* y, double* z, double time) {
// 分解速度为水平、垂直和航向分量
double vx = initial_speed * cos(launch_angle) * cos(heading_angle);
double vy = initial_speed * sin(launch_angle);
double vz = initial_speed * cos(launch_angle) * sin(heading_angle);
// 计算x, y, z坐标
*x = vx * time;
*y = vy * time - 0.5 * g * pow(time, 2);
*z = vz * time + height; // 高度不变,加上初始高度
// 可能需要取模转换为实际距离(如果航向角非零)
// (*z = hypot(*x, *y) * cos(heading_angle) + height;)
}
```
这段代码假设航向角是指向正前方的,所以不需要修正。如果航向角非零,那么最终的z值应该根据航向角调整为实际距离。你可以根据实际情况选择是否进行这种修正。
阅读全文
相关推荐



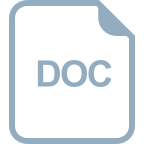














