在ffmpeg中编写一个bitstream filter,针对h264和hevc码流数据进行处理,能够产生各种类型的“问题码流”,如SEI SPS PPS 等数据出错
时间: 2023-06-25 12:07:37 浏览: 68
编写一个 bitstream filter 以处理 H.264 和 HEVC 码流数据,实现产生各种类型的“问题码流”,如 SEI、SPS、PPS 等数据出错,可以按照以下步骤进行:
1. 确定需要产生的“问题码流”类型,如 SEI 数据出错、SPS 数据出错、PPS 数据出错等。
2. 在代码中识别需要产生错误的位置,例如,在 SPS 数据中,可以将 SPS header 中的某些字段设置为不合法的值,或者将 SPS header 中的一些比特翻转。
3. 实现 bitstream filter 的过滤函数,将输入码流数据逐比特解析,遇到需要产生错误的位置时,对数据进行修改,然后再将修改后的数据输出。
4. 编译并使用 bitstream filter 进行测试,检查产生的“问题码流”是否符合预期。
下面是一个简单的示例代码,用于在 H.264 码流中产生 SEI 数据出错:
```c
#include <libavcodec/avcodec.h>
#include <libavutil/opt.h>
typedef struct {
AVBitStreamFilterContext *bsf;
int sei_err;
} MyBitstreamFilterContext;
static int my_filter(AVBitStreamFilterContext *bsfc, AVCodecContext *avctx,
const char *name, AVDictionary **options)
{
MyBitstreamFilterContext *ctx = av_malloc(sizeof(MyBitstreamFilterContext));
if (!ctx) {
return AVERROR(ENOMEM);
}
ctx->bsf = bsfc;
ctx->sei_err = 1;
av_opt_set_int(bsfc, "my_filter", 1, AV_OPT_SEARCH_CHILDREN);
avctx->coded_frame = av_frame_alloc();
if (!avctx->coded_frame) {
av_free(ctx);
return AVERROR(ENOMEM);
}
bsfc->priv_data = ctx;
return 0;
}
static int my_filter_packet(AVBitStreamFilterContext *bsfc, AVPacket *pkt)
{
MyBitstreamFilterContext *ctx = bsfc->priv_data;
uint8_t *data = pkt->data;
int size = pkt->size;
int start_code_found = 0;
while (size > 0) {
uint8_t *next_start_code = avpriv_find_start_code(data, data + size, &start_code_found);
int start_code_size = start_code_found ? 3 : 0;
int nal_type = (next_start_code - data - start_code_size) & 0x1f;
if (nal_type == 6 && ctx->sei_err) {
// SEI 数据出错,将 SEI payload 中的一个比特翻转
uint8_t *sei_payload = next_start_code + 1;
int sei_payload_size = size - (sei_payload - data);
if (sei_payload_size > 0) {
sei_payload[0] ^= 1;
ctx->sei_err = 0;
}
}
size -= next_start_code - data + start_code_size;
data = next_start_code + start_code_size;
}
return 0;
}
AVBitStreamFilter my_filter_bsfs[] = {
{
.name = "my_filter",
.filter = my_filter,
.priv_data_size = sizeof(MyBitstreamFilterContext),
},
{ NULL },
};
int main(int argc, char *argv[])
{
AVBitStreamFilterContext *bsfc = av_bitstream_filter_init("my_filter");
if (!bsfc) {
return 1;
}
av_register_bitstream_filter(my_filter_bsfs);
AVPacket pkt;
av_init_packet(&pkt);
pkt.data = (uint8_t *)"\x00\x00\x01\x06\x01\x02\x03\x04\x05\x06\x07\x08";
pkt.size = 12;
av_bitstream_filter_filter(bsfc, NULL, NULL, &pkt.data, &pkt.size, pkt.data, pkt.size, 0);
// 码流中的 SEI 数据已经被修改
av_bitstream_filter_close(bsfc);
return 0;
}
```
上述代码中,my_filter_packet 函数中的代码实现了在 H.264 码流中产生 SEI 数据出错的逻辑,当 nal_type 等于 6 时,即当前 NALU 为 SEI 数据时,将 SEI payload 中的一个比特翻转。在 main 函数中,通过调用 av_bitstream_filter_filter 函数来应用 bitstream filter,并检查 SEI 数据是否已经被修改。
相关推荐
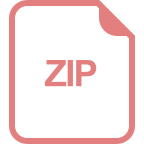
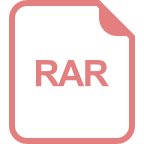







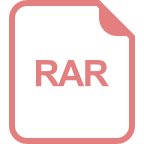
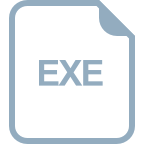
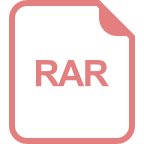
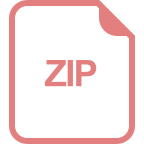
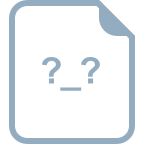
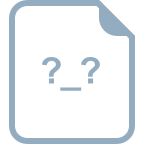