stm32 TLT屏幕 链表上下滚动代码
时间: 2023-06-28 14:10:56 浏览: 157
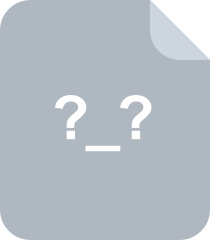
stm32的代码
下面是一个简单的 STM32 上的 TLT 屏幕链表上下滚动的示例代码:
```c
#include "stm32f4xx.h"
#define LINE_HEIGHT 16
#define SCREEN_HEIGHT 128
typedef struct Node {
char* content;
struct Node* next;
} Node;
Node* head = NULL;
uint16_t top_line = 0;
void add_node(char* content);
void scroll_up();
void draw_screen();
int main(void) {
// 初始化 TFT 屏幕
// ...
// 添加测试数据
add_node("Line 1");
add_node("Line 2");
add_node("Line 3");
add_node("Line 4");
add_node("Line 5");
add_node("Line 6");
add_node("Line 7");
add_node("Line 8");
add_node("Line 9");
add_node("Line 10");
add_node("Line 11");
add_node("Line 12");
add_node("Line 13");
add_node("Line 14");
add_node("Line 15");
while (1) {
// 检测是否需要滚动
if (top_line + SCREEN_HEIGHT / LINE_HEIGHT <= count_nodes()) {
scroll_up();
}
// 绘制屏幕
draw_screen();
}
}
void add_node(char* content) {
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->content = content;
new_node->next = NULL;
if (head == NULL) {
head = new_node;
} else {
Node* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = new_node;
}
}
void scroll_up() {
top_line++;
}
void draw_screen() {
// 清空屏幕
// ...
Node* current = head;
uint16_t line = 0;
while (current != NULL && line < top_line) {
current = current->next;
line++;
}
while (current != NULL && line < top_line + SCREEN_HEIGHT / LINE_HEIGHT) {
// 在屏幕上绘制当前行
// ...
current = current->next;
line++;
}
}
uint16_t count_nodes() {
uint16_t count = 0;
Node* current = head;
while (current != NULL) {
count++;
current = current->next;
}
return count;
}
```
在这个示例中,我们使用了一个简单的链表来存储屏幕上的行。当需要滚动时,我们只需要递增 `top_line` 的值,然后重新绘制屏幕即可。注意,在实际代码中,你需要根据你的具体硬件和屏幕库进行适当的调整。
阅读全文
相关推荐
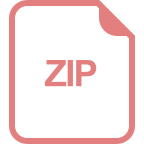



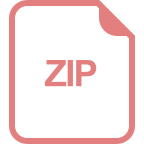


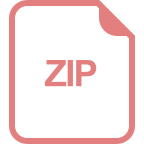
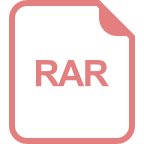
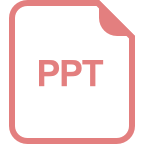
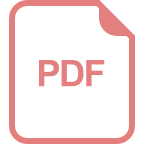


