rsa算法c++语言实现
时间: 2023-09-03 17:15:40 浏览: 70
为了保证数据传输的安全性,RSA算法是一种非常常见的加密算法。在C语言中,可以使用以下代码实现RSA算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/err.h>
#define KEY_LENGTH 2048
#define PUB_EXP 3
int main(int argc, char *argv[]) {
char *pub_key_path = "public.pem"; // 公钥路径
char *pri_key_path = "private.pem"; // 私钥路径
char *plain_text = "Hello, RSA!"; // 明文
char cipher_text[256] = {0}; // 密文
char decrypt_text[256] = {0}; // 解密后的明文
// 1. 生成密钥对
RSA *rsa = RSA_generate_key(KEY_LENGTH, PUB_EXP, NULL, NULL);
if (rsa == NULL) {
printf("RSA_generate_key error.\n");
return -1;
}
// 2. 保存公钥和私钥
FILE *fp = NULL;
fp = fopen(pub_key_path, "wb+");
if (fp == NULL) {
printf("fopen public key error.\n");
return -1;
}
PEM_write_RSAPublicKey(fp, rsa);
fclose(fp);
fp = fopen(pri_key_path, "wb+");
if (fp == NULL) {
printf("fopen private key error.\n");
return -1;
}
PEM_write_RSAPrivateKey(fp, rsa, NULL, NULL, 0, NULL, NULL);
fclose(fp);
// 3. 使用公钥加密
fp = fopen(pub_key_path, "rb");
if (fp == NULL) {
printf("fopen public key error.\n");
return -1;
}
RSA *public_key = RSA_new();
if (public_key == NULL) {
printf("RSA_new public_key error.\n");
return -1;
}
public_key = PEM_read_RSAPublicKey(fp, &public_key, NULL, NULL);
fclose(fp);
int rsa_len = RSA_public_encrypt(strlen(plain_text), (unsigned char *)plain_text, (unsigned char *)cipher_text, public_key, RSA_PKCS1_PADDING);
if (rsa_len == -1) {
printf("RSA_public_encrypt error.\n");
return -1;
}
printf("cipher text: %s\n", cipher_text);
// 4. 使用私钥解密
fp = fopen(pri_key_path, "rb");
if (fp == NULL) {
printf("fopen private key error.\n");
return -1;
}
RSA *private_key = RSA_new();
if (private_key == NULL) {
printf("RSA_new private_key error.\n");
return -1;
}
private_key = PEM_read_RSAPrivateKey(fp, &private_key, NULL, NULL);
fclose(fp);
rsa_len = RSA_private_decrypt(rsa_len, (unsigned char *)cipher_text, (unsigned char *)decrypt_text, private_key, RSA_PKCS1_PADDING);
if (rsa_len == -1) {
printf("RSA_private_decrypt error.\n");
return -1;
}
printf("decrypt text: %s\n", decrypt_text);
return 0;
}
```
注意,在使用该代码时需要安装openssl库。可以使用以下命令在Ubuntu上安装openssl:
```shell
sudo apt-get install libssl-dev
```
相关推荐
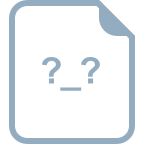
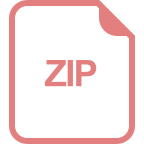
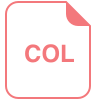













