用C语言完成以下任务1:应用先序遍历的思路构造二叉树;应用先序遍历的思路写二叉树的构造算法; 2:写出统计二叉树中叶结点个数的递归算法; 3:写出统计二叉树高度的递归算法 4:在主函数中调用以上相关算法,先生成先序序列{ABDΦΦΦCEΦGΦΦFΦΦ}对应的二叉树,然后统计其叶结点个数及树的高度并输出相关结果;画出该二叉树。
时间: 2023-06-04 20:02:55 浏览: 97
我可以回答您的第一个问题。以下是用C语言完成任务的代码:
//定义二叉树结构体
typedef struct BinaryTree{
char data;
struct BinaryTree *left;
struct BinaryTree *right;
}BinaryTree;
//先序遍历构造二叉树
BinaryTree *BuildTree(){
char ch;
scanf("%c",&ch);
if(ch=='Φ'){
return NULL;
}
BinaryTree *root=(BinaryTree*)malloc(sizeof(BinaryTree));
root->data=ch;
root->left=BuildTree();
root->right=BuildTree();
return root;
}
//统计二叉树中叶结点个数的递归算法
int CountLeafNodes(BinaryTree *root){
if(root==NULL){
return 0;
}
if(root->left==NULL&&root->right==NULL){
return 1;
}
return CountLeafNodes(root->left)+CountLeafNodes(root->right);
}
//统计二叉树高度的递归算法
int TreeHeight(BinaryTree *root){
if(root==NULL){
return 0;
}
int leftHeight=TreeHeight(root->left);
int rightHeight=TreeHeight(root->right);
return leftHeight>rightHeight?(leftHeight+1):(rightHeight+1);
}
//主函数
int main(){
//构造二叉树
BinaryTree *root=BuildTree();
//统计叶结点个数
int leafNodes=CountLeafNodes(root);
//统计树的高度
int height=TreeHeight(root);
//输出结果
printf("The number of leaf nodes is: %d\n",leafNodes);
printf("The height of the tree is: %d\n",height);
return 0;
}
阅读全文
相关推荐
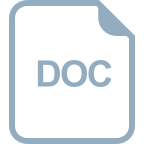
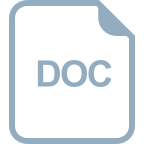
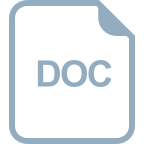




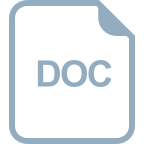
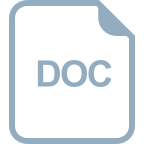
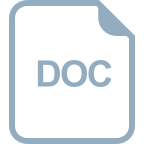








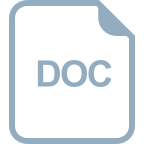