一个 n个点 m 条边的无向图,请求出从 s 到 t 的最短路长度。 输入格式 第一行四个正整数 n, m, s, t。 接下来 m 行,每行三个正整数 u, v, w,表示一条连接 u, v 长为 w 的边。 1≤n≤2500,1≤m≤6200,1≤w≤1000。 输出格式 输出一行一个整数,表示答案。 样例输入 7 11 5 4 2 4 2 1 4 3 7 2 2 3 4 3 5 7 5 7 3 3 6 1 1 6 3 4 2 4 3 5 6 3 7 2 1 样例输出 7用c++实现
时间: 2023-12-29 16:06:06 浏览: 50
好的,以下是使用 Dijkstra 算法的 C++ 实现代码,希望对你有所帮助:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <cstring>
using namespace std;
const int N = 2510, INF = 0x3f3f3f3f;
int n, m, s, t;
vector<pair<int, int>> graph[N];
int dist[N];
bool visited[N];
int dijkstra() {
memset(dist, INF, sizeof(dist));
memset(visited, false, sizeof(visited));
dist[s] = 0;
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<>> pq;
pq.push({0, s});
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
if (visited[u]) continue;
visited[u] = true;
for (auto [v, w] : graph[u]) {
if (!visited[v] && dist[v] > dist[u] + w) {
dist[v] = dist[u] + w;
pq.push({dist[v], v});
}
}
}
return dist[t];
}
int main() {
cin >> n >> m >> s >> t;
for (int i = 0; i < m; i++) {
int u, v, w;
cin >> u >> v >> w;
graph[u].push_back({v, w});
graph[v].push_back({u, w});
}
cout << dijkstra() << endl;
return 0;
}
```
该代码通过邻接表存储图,并使用堆优化的 Dijkstra 算法求解最短路。时间复杂度为 O(mlogn),其中 m 是边数,n 是顶点数。
相关推荐
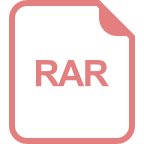
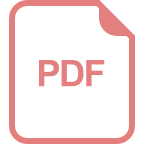















