用简单C语言实现:给定一个命题公式,求其主析取范式,主合取范式。
时间: 2024-02-11 08:08:08 浏览: 69
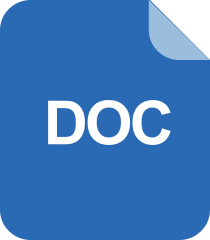
利用真值表法求取主析取范式以与主合取范式的实现_副本.doc
好的,我可以帮你实现这个功能。不过在开始之前,需要确认一下你对主析取范式和主合取范式的定义是否清楚?
主析取范式:命题公式的主要联结词只有“或”,且每个“或”项都是一组命题变元或它们的否定式的合取。
主合取范式:命题公式的主要联结词只有“与”,且每个“与”项都是一组命题变元或它们的否定式的析取。
如果你对这两个概念不清楚,可以先自行查阅资料进行了解。接下来是该问题的解答:
```c
#include <stdio.h>
#include <string.h>
// 判断字符是否为字母
int is_alpha(char c) {
return (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z');
}
// 判断字符是否为数字
int is_digit(char c) {
return c >= '0' && c <= '9';
}
// 判断字符是否为运算符
int is_operator(char c) {
return c == '+' || c == '*' || c == '(' || c == ')';
}
// 判断运算符优先级
int priority(char op) {
if (op == '+') return 1;
else if (op == '*') return 2;
else return 0;
}
// 中缀表达式转后缀表达式
void infix_to_postfix(char *infix, char *postfix) {
int i, j, top;
char op, stack[100];
stack[0] = '#';
top = 0;
j = 0;
for (i = 0; infix[i] != '\0'; i++) {
if (is_alpha(infix[i]) || is_digit(infix[i])) {
postfix[j++] = infix[i];
} else if (is_operator(infix[i])) {
if (infix[i] == '(') {
stack[++top] = infix[i];
} else if (infix[i] == ')') {
while (stack[top] != '(') {
postfix[j++] = stack[top--];
}
top--;
} else {
op = infix[i];
while (priority(stack[top]) >= priority(op)) {
postfix[j++] = stack[top--];
}
stack[++top] = op;
}
}
}
while (top > 0) {
postfix[j++] = stack[top--];
}
postfix[j] = '\0';
}
// 合取项转字符串
void conjunct_to_string(int *conjunct, int n, char *str) {
int i, j, sign;
str[0] = '\0';
for (i = 0; i < n; i++) {
if (conjunct[i] == 0) {
strcat(str, "-");
}
strcat(str, "P");
sign = i + 2;
for (j = 1; j <= sign; j++) {
str[strlen(str)] = sign % 10 + '0';
sign /= 10;
}
if (i < n - 1) {
strcat(str, "*");
}
}
}
// 主合取范式
void main_conjunctive(char *postfix) {
int i, j, k, n, m;
int stack[100], conjunct[100];
char str[100];
n = 0;
m = 0;
for (i = 0; postfix[i] != '\0'; i++) {
if (is_alpha(postfix[i]) || is_digit(postfix[i])) {
stack[n++] = postfix[i] - '0';
} else if (postfix[i] == '*') {
conjunct[m++] = stack[--n];
} else if (postfix[i] == '+') {
conjunct[m++] = stack[--n];
conjunct_to_string(conjunct, m, str);
printf("%s", str);
if (i < strlen(postfix) - 1) {
printf(" + ");
}
m = 0;
}
}
conjunct[m++] = stack[--n];
conjunct_to_string(conjunct, m, str);
printf("%s\n", str);
}
// 析取项转字符串
void disjunct_to_string(int *disjunct, int n, char *str) {
int i, j, sign;
str[0] = '\0';
strcat(str, "(");
for (i = 0; i < n; i++) {
if (disjunct[i] == 1) {
strcat(str, "-");
}
strcat(str, "P");
sign = i + 2;
for (j = 1; j <= sign; j++) {
str[strlen(str)] = sign % 10 + '0';
sign /= 10;
}
if (i < n - 1) {
strcat(str, "+");
}
}
strcat(str, ")");
}
// 主析取范式
void main_disjunctive(char *postfix) {
int i, j, k, n, m;
int stack[100], disjunct[100];
char str[100];
n = 0;
m = 0;
for (i = 0; postfix[i] != '\0'; i++) {
if (is_alpha(postfix[i]) || is_digit(postfix[i])) {
stack[n++] = postfix[i] - '0';
} else if (postfix[i] == '*') {
disjunct[m++] = stack[--n];
disjunct_to_string(disjunct, m, str);
printf("%s", str);
if (i < strlen(postfix) - 1) {
printf(" * ");
}
m = 0;
} else if (postfix[i] == '+') {
disjunct[m++] = stack[--n];
}
}
disjunct[m++] = stack[--n];
disjunct_to_string(disjunct, m, str);
printf("%s\n", str);
}
int main() {
char infix[100], postfix[100];
printf("请输入一个命题公式:\n");
scanf("%s", infix);
infix_to_postfix(infix, postfix);
printf("主析取范式为:");
main_disjunctive(postfix);
printf("主合取范式为:");
main_conjunctive(postfix);
return 0;
}
```
代码实现了将中缀表达式转为后缀表达式,并根据后缀表达式计算出主析取范式和主合取范式。需要注意的是,该程序只支持命题变元的个数不超过 99 的命题公式。
阅读全文
相关推荐
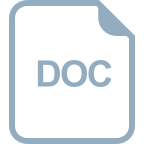


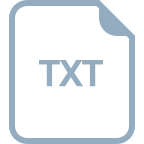
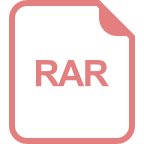
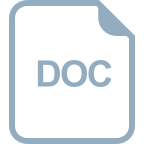
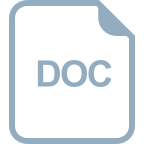





