单链表如何在输入非整数后重新输入c++
时间: 2024-02-20 20:01:14 浏览: 51
在C++中,可以使用循环和异常处理来检测并重新输入用户的非整数输入。以下是一个示例代码:
```c++
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node* next;
Node(int value) : data(value), next(nullptr) {}
};
class LinkedList {
public:
Node* head;
LinkedList() : head(nullptr) {}
void insert(int value) {
if (!head) {
head = new Node(value);
}
else {
Node* current = head;
while (current->next) {
current = current->next;
}
current->next = new Node(value);
}
}
void input_integer() {
while (true) {
try {
int value;
cout << "请输入一个整数:";
cin >> value;
insert(value);
break;
}
catch (...) {
cout << "输入有误,请重新输入!" << endl;
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
}
}
}
void print_list() {
Node* current = head;
while (current) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
}
};
int main() {
LinkedList linked_list;
for (int i = 0; i < 5; i++) {
linked_list.input_integer();
}
linked_list.print_list();
return 0;
}
```
在这个示例代码中,`input_integer` 方法用于接收用户输入的整数,并将其插入到单链表中。如果用户输入的是非整数,就会提示错误并要求用户重新输入。`print_list` 方法用于打印单链表中的所有元素。这个示例代码中在异常处理时,使用了 `cin.clear()` 和 `cin.ignore()` 来清除输入流和忽略输入流中的字符。
阅读全文
相关推荐
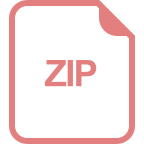




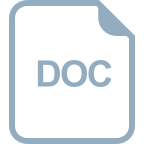










