用c语言实现图的基本存储,图的邻接矩阵存储(C语言实现)
时间: 2024-02-21 14:00:30 浏览: 75
实现图的邻接矩阵存储需要定义一个结构体来表示图,其中包含顶点数和邻接矩阵的二维数组。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTEX_NUM 20
// 定义图的结构体
typedef struct {
char vertex[MAX_VERTEX_NUM]; // 顶点数组
int edges[MAX_VERTEX_NUM][MAX_VERTEX_NUM]; // 邻接矩阵
int vertex_num; // 顶点数
int edge_num; // 边数
} Graph;
// 初始化图
void init_graph(Graph *G, int vertex_num) {
int i, j;
G->vertex_num = vertex_num;
G->edge_num = 0;
for (i = 0; i < G->vertex_num; i++) {
for (j = 0; j < G->vertex_num; j++) {
G->edges[i][j] = 0;
}
}
}
// 添加边
void add_edge(Graph *G, int v1, int v2) {
G->edges[v1][v2] = 1;
G->edges[v2][v1] = 1;
G->edge_num++;
}
// 打印图
void print_graph(Graph *G) {
int i, j;
printf("顶点数:%d,边数:%d\n", G->vertex_num, G->edge_num);
for (i = 0; i < G->vertex_num; i++) {
for (j = 0; j < G->vertex_num; j++) {
printf("%d ", G->edges[i][j]);
}
printf("\n");
}
}
int main() {
Graph G;
init_graph(&G, 5);
add_edge(&G, 0, 1);
add_edge(&G, 0, 2);
add_edge(&G, 1, 2);
add_edge(&G, 1, 3);
add_edge(&G, 2, 4);
print_graph(&G);
return 0;
}
```
在此代码中,我们定义了一个结构体 `Graph` 来表示图,其中包含顶点数组、邻接矩阵和顶点数、边数等信息。我们使用 `init_graph` 函数来初始化图,将其顶点数设置为 `vertex_num`,然后将邻接矩阵中的每个元素初始化为 0。使用 `add_edge` 函数来添加边,将邻接矩阵中对应的元素设置为 1,同时边数加 1。最后使用 `print_graph` 函数来打印图的信息。
阅读全文
相关推荐














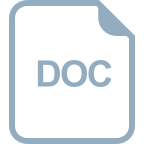
