c语言实现有向图的邻接矩阵存储
时间: 2023-09-04 11:10:45 浏览: 113
有向图的邻接矩阵存储是指用一个二维数组来表示图中各个顶点之间的关系,如果顶点i到j有一条有向边,则数组中第i行第j列的元素为1,否则为0。以下是C语言实现有向图邻接矩阵存储的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTEX_NUM 20 // 最大顶点数
// 定义有向图结构体
typedef struct {
int vertex[MAX_VERTEX_NUM]; // 存储顶点数据
int edge[MAX_VERTEX_NUM][MAX_VERTEX_NUM]; // 存储边的关系
int vertex_num; // 顶点数
int edge_num; // 边数
} DirectedGraph;
// 初始化有向图
void initDirectedGraph(DirectedGraph* graph) {
int i, j;
graph->vertex_num = 0;
graph->edge_num = 0;
for (i = 0; i < MAX_VERTEX_NUM; i++) {
graph->vertex[i] = 0;
for (j = 0; j < MAX_VERTEX_NUM; j++) {
graph->edge[i][j] = 0;
}
}
}
// 添加顶点
void addVertex(DirectedGraph* graph, int vertex) {
if (graph->vertex_num == MAX_VERTEX_NUM) {
printf("The graph is full, cannot add vertex.\n");
return;
}
graph->vertex[graph->vertex_num++] = vertex;
}
// 添加边
void addEdge(DirectedGraph* graph, int start, int end) {
int i, start_index = -1, end_index = -1;
for (i = 0; i < graph->vertex_num; i++) {
if (graph->vertex[i] == start) {
start_index = i;
}
if (graph->vertex[i] == end) {
end_index = i;
}
}
if (start_index == -1 || end_index == -1) {
printf("Vertex not found, cannot add edge.\n");
return;
}
if (graph->edge[start_index][end_index] == 1) {
printf("The edge already exists.\n");
return;
}
graph->edge[start_index][end_index] = 1;
graph->edge_num++;
}
// 打印有向图
void printDirectedGraph(DirectedGraph* graph) {
int i, j;
printf("The directed graph has %d vertex(es) and %d edge(s).\n", graph->vertex_num, graph->edge_num);
printf("Vertexes: ");
for (i = 0; i < graph->vertex_num; i++) {
printf("%d ", graph->vertex[i]);
}
printf("\n");
printf("Edges:\n");
for (i = 0; i < graph->vertex_num; i++) {
for (j = 0; j < graph->vertex_num; j++) {
printf("%d ", graph->edge[i][j]);
}
printf("\n");
}
}
int main() {
DirectedGraph graph;
initDirectedGraph(&graph);
addVertex(&graph, 1);
addVertex(&graph, 2);
addVertex(&graph, 3);
addVertex(&graph, 4);
addEdge(&graph, 1, 2);
addEdge(&graph, 1, 3);
addEdge(&graph, 2, 4);
addEdge(&graph, 3, 4);
printDirectedGraph(&graph);
return 0;
}
```
输出结果为:
```
The directed graph has 4 vertex(es) and 4 edge(s).
Vertexes: 1 2 3 4
Edges:
0 1 1 0
0 0 0 1
0 0 0 1
0 0 0 0
```
阅读全文
相关推荐
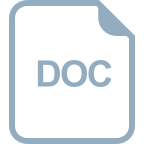
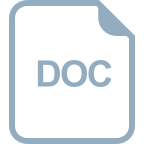
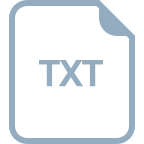
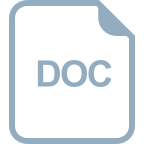
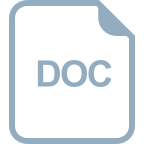
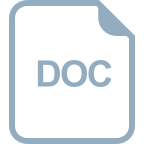









