帮我实现一个canvas绘制多边形的vue组件
时间: 2024-06-11 15:05:17 浏览: 141
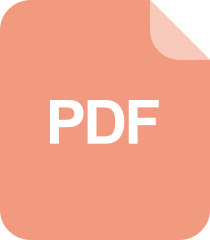
canvas绘制多边形
以下是一个简单的多边形绘制组件的Vue代码,它使用Canvas API绘制多边形:
```html
<template>
<div>
<canvas ref="canvas" @mousedown="startDrawing" @mousemove="draw" @mouseup="endDrawing"></canvas>
</div>
</template>
<script>
export default {
name: 'PolygonDrawing',
props: {
sides: {
type: Number,
default: 5
},
radius: {
type: Number,
default: 100
},
color: {
type: String,
default: '#000000'
}
},
mounted() {
this.canvas = this.$refs.canvas;
this.ctx = this.canvas.getContext('2d');
this.drawPolygon();
},
methods: {
drawPolygon() {
const centerX = this.canvas.width / 2;
const centerY = this.canvas.height / 2;
const angle = (2 * Math.PI) / this.sides;
this.ctx.beginPath();
this.ctx.moveTo(centerX + this.radius, centerY);
for (let i = 1; i <= this.sides; i++) {
const x = centerX + this.radius * Math.cos(i * angle);
const y = centerY + this.radius * Math.sin(i * angle);
this.ctx.lineTo(x, y);
}
this.ctx.strokeStyle = this.color;
this.ctx.stroke();
},
startDrawing(event) {
this.isDrawing = true;
this.ctx.beginPath();
this.ctx.moveTo(event.offsetX, event.offsetY);
},
draw(event) {
if (!this.isDrawing) return;
this.ctx.lineTo(event.offsetX, event.offsetY);
this.ctx.strokeStyle = this.color;
this.ctx.stroke();
},
endDrawing() {
this.isDrawing = false;
}
},
watch: {
sides() {
this.ctx.clearRect(0, 0, this.canvas.width, this.canvas.height);
this.drawPolygon();
},
radius() {
this.ctx.clearRect(0, 0, this.canvas.width, this.canvas.height);
this.drawPolygon();
},
color() {
this.ctx.clearRect(0, 0, this.canvas.width, this.canvas.height);
this.drawPolygon();
}
}
};
</script>
```
该组件包含三个 props:
- `sides`:多边形的边数,默认为 5。
- `radius`:多边形的半径,默认为 100。
- `color`:绘制多边形和边的颜色,默认为黑色。
在 `mounted` 钩子中,我们获取到 `canvas` 元素和它的 2D 上下文对象 `ctx`。然后我们调用 `drawPolygon` 方法来绘制一个多边形。
`drawPolygon` 方法中,我们首先计算多边形的中心点坐标,然后使用 `moveTo` 和 `lineTo` 方法绘制多边形的每个顶点之间的线段,最后使用 `stroke` 方法来绘制多边形的边框。
在鼠标事件处理函数中,我们使用 `isDrawing` 状态来标记是否正在绘制。在 `startDrawing` 中,我们开始新的路径并将它的起点设为鼠标点击的位置。在 `draw` 中,如果正在绘制,我们使用 `lineTo` 方法来连接当前鼠标位置和上一个鼠标位置,并使用 `stroke` 方法绘制线段。在 `endDrawing` 中,我们将 `isDrawing` 状态重置为 `false`,表示绘制已经结束。
最后,我们使用 `watch` 监听器来监测 props 的变化,并在变化时重新绘制多边形。
阅读全文
相关推荐
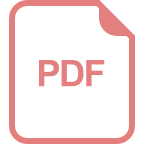


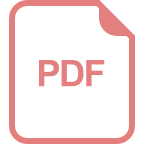
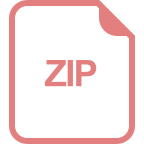
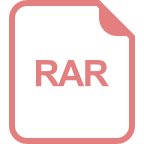
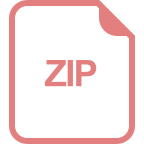
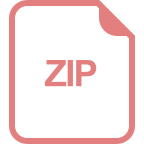







