vue的canvas绘制多个多边形并添加点击事件
时间: 2024-02-15 20:54:01 浏览: 334
要在Vue中使用canvas绘制多个多边形并添加点击事件,可以按照以下步骤操作:
1. 在Vue组件中创建canvas元素,并设置其宽度和高度:
```
<template>
<div>
<canvas ref="canvas" width="400" height="400"></canvas>
</div>
</template>
```
2. 在组件的mounted钩子函数中获取canvas元素和其上下文对象:
```
<script>
export default {
mounted() {
this.canvas = this.$refs.canvas;
this.ctx = this.canvas.getContext('2d');
}
}
</script>
```
3. 在组件中定义一个数组,用于存储多边形的顶点坐标:
```
data() {
return {
polygons: [
[{x: 50, y: 50}, {x: 100, y: 50}, {x: 75, y: 75}],
[{x: 150, y: 150}, {x: 200, y: 150}, {x: 175, y: 175}],
[{x: 250, y: 250}, {x: 300, y: 250}, {x: 275, y: 275}]
]
}
}
```
4. 在组件中定义一个方法,用于绘制所有的多边形:
```
methods: {
drawPolygons() {
this.ctx.clearRect(0, 0, this.canvas.width, this.canvas.height);
this.polygons.forEach(points => {
this.ctx.beginPath();
this.ctx.moveTo(points[0].x, points[0].y);
points.forEach(p => {
this.ctx.lineTo(p.x, p.y);
});
this.ctx.closePath();
this.ctx.stroke();
});
}
}
```
5. 在组件的mounted钩子函数中调用drawPolygons方法,以绘制多边形:
```
mounted() {
this.canvas = this.$refs.canvas;
this.ctx = this.canvas.getContext('2d');
this.drawPolygons();
}
```
6. 在canvas元素上添加鼠标点击事件,以便在多边形上单击时触发:
```
mounted() {
this.canvas = this.$refs.canvas;
this.ctx = this.canvas.getContext('2d');
this.drawPolygons();
this.canvas.addEventListener('click', this.onCanvasClick);
},
methods: {
onCanvasClick(event) {
const x = event.offsetX;
const y = event.offsetY;
this.polygons.forEach(points => {
this.ctx.beginPath();
this.ctx.moveTo(points[0].x, points[0].y);
points.forEach(p => {
this.ctx.lineTo(p.x, p.y);
});
this.ctx.closePath();
if (this.ctx.isPointInPath(x, y)) {
console.log('clicked polygon');
// do something when polygon is clicked
}
});
},
// ...
}
```
这样,就可以使用Vue和canvas绘制多个多边形,并添加点击事件了。
阅读全文
相关推荐
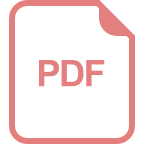

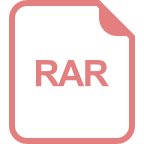
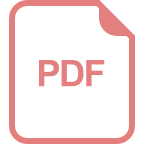







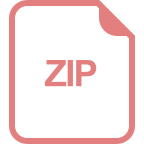
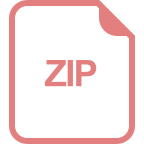
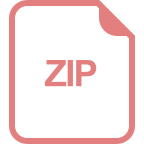
