假设二叉树每个结点的元素均为一个单字符,根据给定的字符序列按照先序遍历的顺序递归创建该树的二叉链表,然后判断该二叉树是否为二叉排序树。用C语言
时间: 2024-05-10 12:17:10 浏览: 18
以下是C语言的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
char val;
struct TreeNode* left;
struct TreeNode* right;
};
// 递归创建二叉树
struct TreeNode* createTree(char* str, int* index) {
if (str[*index] == '#') {
*index += 1;
return NULL;
}
struct TreeNode* node = (struct TreeNode*)malloc(sizeof(struct TreeNode));
node->val = str[*index];
*index += 1;
node->left = createTree(str, index);
node->right = createTree(str, index);
return node;
}
// 判断是否为二叉排序树
int isBST(struct TreeNode* root, char min, char max) {
if (root == NULL) {
return 1; // 空树也是二叉排序树
}
if (root->val < min || root->val > max) {
return 0; // 当前节点不在[min, max]范围内
}
return isBST(root->left, min, root->val) && isBST(root->right, root->val, max);
}
int main() {
char str[] = "DBACE##F##G##";
int index = 0;
struct TreeNode* root = createTree(str, &index);
if (isBST(root, '\0', '\xFF')) {
printf("The binary tree is a binary search tree.\n");
} else {
printf("The binary tree is not a binary search tree.\n");
}
return 0;
}
```
其中,`createTree`函数用于根据给定的字符序列按照先序遍历的顺序递归创建二叉树;`isBST`函数用于判断该二叉树是否为二叉排序树。在`isBST`函数中,用`min`和`max`表示当前节点值的取值范围,若当前节点不在该范围内,则说明不是二叉排序树。最后在`main`函数中调用`createTree`函数创建二叉树,并调用`isBST`函数判断是否为二叉排序树。
相关推荐
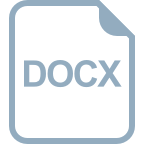
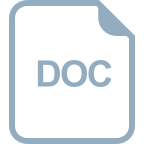
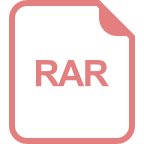








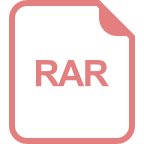
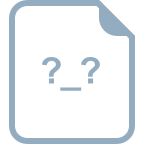
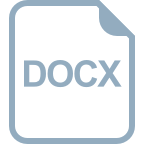
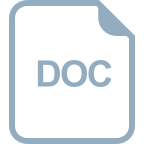
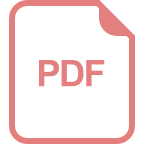
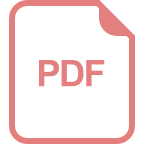
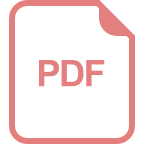
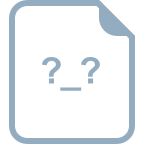