搭建一个基于pytorch和DGL的gcn模型,并给出完整代码
时间: 2023-02-14 07:29:33 浏览: 151
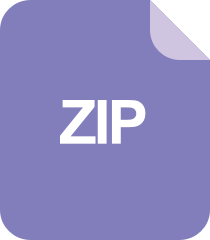
基于pytorch框架的深层CNN
在 PyTorch 中搭建一个基于 DGL 的 GCN 模型,需要以下步骤:
1. 安装 DGL 库: `pip install dgl`
2. 导入 DGL 中 GCN 模型所需的库:
```python
import dgl
import dgl.function as fn
import torch
import torch.nn as nn
```
3. 定义 GCNLayer 类继承自 nn.Module,并实现 forward() 函数:
```python
class GCNLayer(nn.Module):
def __init__(self, in_feats, out_feats):
super(GCNLayer, self).__init__()
self.linear = nn.Linear(in_feats, out_feats)
def forward(self, g, inputs):
# g is the graph and the inputs is the input node features
# first set the node features
g.ndata['h'] = inputs
# then send the node features to its neighbors
g.update_all(fn.copy_src('h', 'm'), fn.sum('m', 'h'))
# apply linear transformation
h = self.linear(g.ndata['h'])
return h
```
4. 构建 GCN 模型,并定义损失函数和优化器:
```python
class GCN(nn.Module):
def __init__(self, in_feats, hidden_size, num_classes):
super(GCN, self).__init__()
self.layer1 = GCNLayer(in_feats, hidden_size)
self.layer2 = GCNLayer(hidden_size, num_classes)
def forward(self, g, inputs):
h = self.layer1(g, inputs)
h = torch.relu(h)
h = self.layer2(g, h)
return h
# define loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
```
5. 加载数据并训练模型:
```python
# load your data
g, inputs, labels = ...
# training loop
for epoch in range(100):
logits = model(g, inputs)
loss = criterion(logits, labels)
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
以上是一个简单的 GCN 模型的实现,你可以根据你的数据和需求来修改
阅读全文
相关推荐
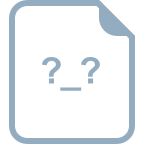
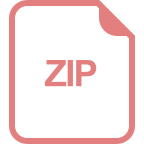
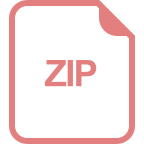
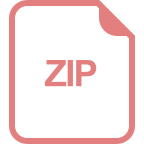
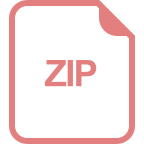
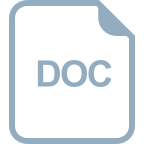
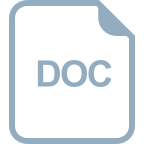
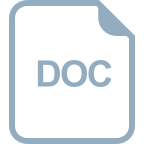
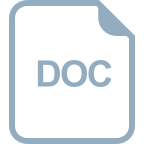
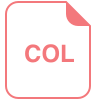






