可以用库函数写吗
时间: 2023-08-15 15:08:15 浏览: 35
当然可以,以下是一个使用STM32 HAL库函数实现按键控制数字加减的示例代码。在该代码中,使用PA0和PA1两个GPIO口连接按键,用于控制数字的加减,数字的初始值为499:
```
#include "main.h"
#include "stdio.h"
#include "string.h"
#define MAX_NUM 499
#define MIN_NUM 0
int get_num(int button1_state, int button2_state, int current_num);
int main(void)
{
HAL_Init();
__HAL_RCC_GPIOA_CLK_ENABLE();
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.Pin = GPIO_PIN_0 | GPIO_PIN_1;
GPIO_InitStruct.Mode = GPIO_MODE_INPUT;
GPIO_InitStruct.Pull = GPIO_PULLUP;
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
int num = MAX_NUM;
while (1)
{
// 读取按键状态
int button1_state = HAL_GPIO_ReadPin(GPIOA, GPIO_PIN_0);
int button2_state = HAL_GPIO_ReadPin(GPIOA, GPIO_PIN_1);
// 更新数字
num = get_num(button1_state, button2_state, num);
// 显示当前数字
char disp_str[50];
sprintf(disp_str, "Current number: %d\n", num);
HAL_UART_Transmit(&huart2, (uint8_t*)disp_str, strlen(disp_str), HAL_MAX_DELAY);
HAL_Delay(100);
}
}
int get_num(int button1_state, int button2_state, int current_num)
{
// 减少数字
if(button1_state == GPIO_PIN_RESET)
{
current_num -= 10;
if(current_num < MIN_NUM)
{
current_num = MAX_NUM;
}
}
// 增加数字
if(button2_state == GPIO_PIN_RESET)
{
current_num += 10;
if(current_num > MAX_NUM)
{
current_num = MIN_NUM;
}
}
return current_num;
}
```
在上面的代码中,使用HAL_GPIO_Init()函数来初始化PA0和PA1两个GPIO口为输入模式,并启用上拉电阻。然后在主循环中使用HAL_GPIO_ReadPin()函数读取按键状态,并使用get_num()函数来根据按键状态更新数字的值。数字的当前值通过get_num()函数返回,并在主循环中显示在串口中。
相关推荐
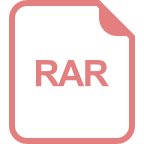
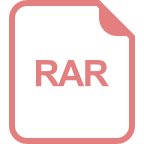
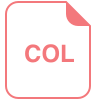
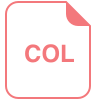












