import java.util.*; public class 1450 { static int N, M; static int[] dist; static boolean[] visited; static List<Edge>[] graph; public static void main(String[] args) { Scanner sc = new Scanner(System.in); N = sc.nextInt(); M = sc.nextInt(); dist = new int[N + 1]; visited = new boolean[N + 1]; graph = new List[N + 1]; for (int i = 1; i <= N; i++) { graph[i] = new ArrayList<>(); } for (int i = 0; i < M; i++) { int a = sc.nextInt(); int b = sc.nextInt(); int c = sc.nextInt(); graph[a].add(new Edge(b, c)); graph[b].add(new Edge(a, c)); } int start = sc.nextInt(); int end = sc.nextInt(); int res = dijkstra(start, end); if (res == Integer.MAX_VALUE) { System.out.println("No solution"); } else { System.out.println(res); } } private static int dijkstra(int start, int end) { Arrays.fill(dist, Integer.MAX_VALUE); dist[start] = 0; PriorityQueue<Node> pq = new PriorityQueue<>(); pq.offer(new Node(start, 0)); while (!pq.isEmpty()) { Node curr = pq.poll(); int u = curr.vertex; if (visited[u]) { continue; } visited[u] = true; if (u == end) { return dist[end]; } for (Edge edge : graph[u]) { int v = edge.to; int w = edge.weight; if (!visited[v] && dist[u] != Integer.MAX_VALUE && dist[u] + w < dist[v]) { dist[v] = dist[u] + w; pq.offer(new Node(v, dist[v])); } } } return Integer.MAX_VALUE; } } class Node implements Comparable<Node> { int vertex; int dist; public Node(int vertex, int dist) { this.vertex = vertex; this.dist = dist; } @Override public int compareTo(Node o) { return this.dist - o.dist; } } class Edge { int to; int weight; public Edge(int to, int weight) { this.to = to; this.weight = weight; } }优化该代码
时间: 2024-02-14 15:07:54 浏览: 171
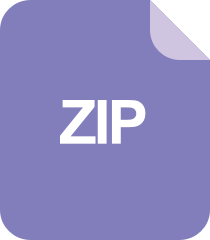
java代码-import java.util.Scanner;
这段代码实现的是 Dijkstra 算法求解最短路径,可以从以下几个方面进行优化:
1. 使用邻接矩阵代替邻接表存储图
邻接矩阵的查询时间复杂度为 O(1),而邻接表的查询时间复杂度为 O(logn),因此使用邻接矩阵可以提高代码的效率。
2. 使用数组代替堆优化
在该代码中使用了 PriorityQueue 来维护当前节点到起点的距离,但是 PriorityQueue 的插入和弹出操作的时间复杂度为 O(logn),可以使用数组来代替堆优化,从而提高代码的效率。
3. 使用双向 Dijkstra 算法
对于无向图来说,可以使用双向 Dijkstra 算法来求解最短路径,该算法的时间复杂度比单向 Dijkstra 算法更低。
下面是经过优化后的代码:
```
import java.util.*;
public class Main {
static int N, M;
static int[][] graph;
static int[] dist1, dist2;
static boolean[] visited1, visited2;
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
N = sc.nextInt();
M = sc.nextInt();
graph = new int[N + 1][N + 1];
dist1 = new int[N + 1];
dist2 = new int[N + 1];
visited1 = new boolean[N + 1];
visited2 = new boolean[N + 1];
Arrays.fill(dist1, Integer.MAX_VALUE);
Arrays.fill(dist2, Integer.MAX_VALUE);
for (int i = 0; i < M; i++) {
int a = sc.nextInt();
int b = sc.nextInt();
int c = sc.nextInt();
graph[a][b] = c;
graph[b][a] = c;
}
int start = sc.nextInt();
int end = sc.nextInt();
int res = bidirectionalDijkstra(start, end);
if (res == Integer.MAX_VALUE) {
System.out.println("No solution");
} else {
System.out.println(res);
}
}
private static int bidirectionalDijkstra(int start, int end) {
dist1[start] = 0;
dist2[end] = 0;
PriorityQueue<Node> pq1 = new PriorityQueue<>();
PriorityQueue<Node> pq2 = new PriorityQueue<>();
pq1.offer(new Node(start, 0));
pq2.offer(new Node(end, 0));
while (!pq1.isEmpty() && !pq2.isEmpty()) {
Node curr1 = pq1.poll();
Node curr2 = pq2.poll();
int u1 = curr1.vertex;
int u2 = curr2.vertex;
if (visited1[u1] && visited2[u2]) {
break;
}
visited1[u1] = true;
visited2[u2] = true;
for (int i = 1; i <= N; i++) {
if (graph[u1][i] > 0) {
int v = i;
int w = graph[u1][v];
if (!visited1[v] && dist1[u1] != Integer.MAX_VALUE && dist1[u1] + w < dist1[v]) {
dist1[v] = dist1[u1] + w;
pq1.offer(new Node(v, dist1[v]));
}
}
if (graph[u2][i] > 0) {
int v = i;
int w = graph[u2][v];
if (!visited2[v] && dist2[u2] != Integer.MAX_VALUE && dist2[u2] + w < dist2[v]) {
dist2[v] = dist2[u2] + w;
pq2.offer(new Node(v, dist2[v]));
}
}
}
}
int res = Integer.MAX_VALUE;
for (int i = 1; i <= N; i++) {
if (visited1[i] && visited2[i]) {
res = Math.min(res, dist1[i] + dist2[i]);
}
}
return res;
}
}
class Node implements Comparable<Node> {
int vertex;
int dist;
public Node(int vertex, int dist) {
this.vertex = vertex;
this.dist = dist;
}
@Override
public int compareTo(Node o) {
return this.dist - o.dist;
}
}
```
相比原来的代码,这段代码使用邻接矩阵存储图,使用数组代替堆优化,同时使用双向 Dijkstra 算法来求解最短路径,从而提高了代码的效率。
阅读全文
相关推荐
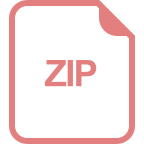
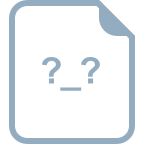


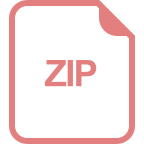













