给一个n(1≤n≤2500)个点m(1≤m≤6200)条边的无向图,求s到t的最短路。用java做
时间: 2024-05-21 10:10:39 浏览: 215
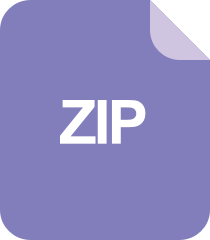
无向图最短路径
以下是Java代码实现,使用了Dijkstra算法:
import java.util.*;
public class ShortestPath {
static class Edge {
int to;
int weight;
Edge(int to, int weight) {
this.to = to;
this.weight = weight;
}
}
static int INF = Integer.MAX_VALUE / 2; // 无穷大
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int n = scanner.nextInt(); // 点的数量
int m = scanner.nextInt(); // 边的数量
List<List<Edge>> graph = new ArrayList<>();
for (int i = 0; i < n; i++) {
graph.add(new ArrayList<>());
}
for (int i = 0; i < m; i++) {
int from = scanner.nextInt() - 1; // 编号从0开始
int to = scanner.nextInt() - 1;
int weight = scanner.nextInt();
graph.get(from).add(new Edge(to, weight));
graph.get(to).add(new Edge(from, weight)); // 无向图
}
int s = scanner.nextInt() - 1; // 起点
int t = scanner.nextInt() - 1; // 终点
int[] dist = new int[n]; // 存储最短路
Arrays.fill(dist, INF); // 初始化为无穷大
dist[s] = 0; // 起点到自身的距离为0
boolean[] visited = new boolean[n]; // 标记是否访问过
PriorityQueue<Integer> pq = new PriorityQueue<>(Comparator.comparingInt(i -> dist[i])); // 优先队列,按照距离从小到大排序
pq.offer(s);
while (!pq.isEmpty()) {
int u = pq.poll();
if (visited[u]) {
continue; // 如果已经访问过,跳过
}
visited[u] = true;
for (Edge e : graph.get(u)) {
int v = e.to;
int weight = e.weight;
if (dist[u] + weight < dist[v]) {
dist[v] = dist[u] + weight;
pq.offer(v);
}
}
}
System.out.println(dist[t] == INF ? -1 : dist[t]); // 如果无法到达终点,输出-1
}
}
阅读全文
相关推荐














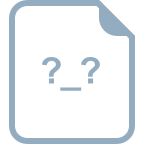
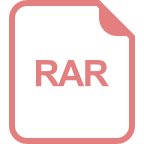