用c语言写一个大鱼吃小鱼的游戏,要有音乐
时间: 2024-04-21 14:29:26 浏览: 170
为了实现大鱼吃小鱼的游戏,我们需要使用到以下技术:
1. 图形界面:使用C语言中的图形库,如graphics.h等;
2. 键盘控制:使用C语言中的键盘输入函数,如getch()等;
3. 碰撞检测:使用检测两个物体之间是否相交的算法,如矩形碰撞检测等;
4. 随机数生成:使用C语言中的随机数函数,如rand()等;
5. 排行榜:使用文件读写操作,将玩家得分保存到文件中,并读取文件中的排行榜信息;
6. 音乐:使用C语言中的音乐库,如mmsystem.h等。
下面是一个简单的示例代码,可供参考:
```
#include <stdio.h>
#include <stdlib.h>
#include <graphics.h>
#include <conio.h>
#include <time.h>
#include <math.h>
#include <Windows.h>
#include <mmsystem.h>
#pragma comment(lib, "winmm.lib")
// 定义窗口大小
#define WIN_WIDTH 800
#define WIN_HEIGHT 600
// 定义鱼的大小和速度
#define FISH_SIZE 80
#define FISH_SPEED 8
// 定义小鱼的数量和大小
#define SMALLFISH_NUM 20
#define SMALLFISH_SIZE 40
// 定义游戏音乐
#define BGM_FILE "bgm.mp3"
#define EAT_FILE "eat.wav"
// 定义鱼和小鱼的结构体
typedef struct {
int x;
int y;
int size;
int speed;
int life;
} Fish;
typedef struct {
int x;
int y;
int size;
int speed;
int alive;
} SmallFish;
// 定义排行榜的结构体
typedef struct {
char name[20];
int score;
} Score;
// 声明函数
void init();
void draw();
void update();
void check_collision();
void generate_smallfish();
void play_bgm();
void play_eat_sound();
void save_score(int score, char* name);
void show_rank();
// 全局变量
Fish fish;
SmallFish smallfish[SMALLFISH_NUM];
int score = 0;
char name[20];
Score rank[10];
// 主函数
int main() {
init();
while (1) {
draw();
update();
check_collision();
delay(50);
}
return 0;
}
// 初始化函数
void init() {
// 初始化窗口
initwindow(WIN_WIDTH, WIN_HEIGHT, "BigFishEatSmallFish");
// 隐藏光标
HideCursor();
// 初始化鱼和小鱼
fish.x = WIN_WIDTH / 2;
fish.y = WIN_HEIGHT / 2;
fish.size = FISH_SIZE;
fish.speed = FISH_SPEED;
fish.life = 3;
srand((unsigned)time(NULL));
for (int i = 0; i < SMALLFISH_NUM; i++) {
smallfish[i].x = rand() % (WIN_WIDTH - SMALLFISH_SIZE);
smallfish[i].y = rand() % (WIN_HEIGHT - SMALLFISH_SIZE);
smallfish[i].size = SMALLFISH_SIZE;
smallfish[i].speed = rand() % 5 + 1;
smallfish[i].alive = 1;
}
// 初始化排行榜
for (int i = 0; i < 10; i++) {
strcpy(rank[i].name, " ");
rank[i].score = 0;
}
}
// 绘制函数
void draw() {
// 清空窗口
cleardevice();
// 绘制鱼
setcolor(YELLOW);
setfillstyle(SOLID_FILL, YELLOW);
fillellipse(fish.x, fish.y, fish.size / 2, fish.size / 2);
// 绘制小鱼
setcolor(GREEN);
setfillstyle(SOLID_FILL, GREEN);
for (int i = 0; i < SMALLFISH_NUM; i++) {
if (smallfish[i].alive) {
fillellipse(smallfish[i].x, smallfish[i].y, smallfish[i].size / 2, smallfish[i].size / 2);
}
}
// 绘制得分和生命值
settextstyle(20, 0, "宋体");
char score_str[20];
sprintf(score_str, "得分:%d", score);
outtextxy(10, 10, score_str);
char life_str[20];
sprintf(life_str, "生命值:%d", fish.life);
outtextxy(10, 40, life_str);
}
// 更新函数
void update() {
// 移动鱼
int key = getch();
switch (key) {
case 72: // 上
fish.y -= fish.speed;
if (fish.y < 0) {
fish.y = 0;
}
break;
case 80: // 下
fish.y += fish.speed;
if (fish.y > WIN_HEIGHT) {
fish.y = WIN_HEIGHT;
}
break;
case 75: // 左
fish.x -= fish.speed;
if (fish.x < 0) {
fish.x = 0;
}
break;
case 77: // 右
fish.x += fish.speed;
if (fish.x > WIN_WIDTH) {
fish.x = WIN_WIDTH;
}
break;
default:
break;
}
// 移动小鱼
for (int i = 0; i < SMALLFISH_NUM; i++) {
if (smallfish[i].alive) {
smallfish[i].x += smallfish[i].speed;
if (smallfish[i].x > WIN_WIDTH) {
smallfish[i].x = 0;
smallfish[i].y = rand() % (WIN_HEIGHT - SMALLFISH_SIZE);
smallfish[i].size = SMALLFISH_SIZE;
smallfish[i].speed = rand() % 5 + 1;
}
}
}
}
// 碰撞检测函数
void check_collision() {
// 检测鱼和小鱼是否相撞
for (int i = 0; i < SMALLFISH_NUM; i++) {
if (smallfish[i].alive) {
int dist = sqrt(pow(smallfish[i].x - fish.x, 2) + pow(smallfish[i].y - fish.y, 2));
if (dist < (smallfish[i].size / 2 + fish.size / 2)) {
smallfish[i].alive = 0;
score++;
play_eat_sound();
}
}
}
// 检测鱼是否死亡
if (score % 10 == 0 && fish.life < 3) {
fish.life++;
}
if (fish.life == 0) {
// 保存得分并显示排行榜
save_score(score, name);
show_rank();
// 重新开始游戏
init();
}
}
// 生成小鱼
void generate_smallfish() {
for (int i = 0; i < SMALLFISH_NUM; i++) {
if (!smallfish[i].alive) {
smallfish[i].x = rand() % (WIN_WIDTH - SMALLFISH_SIZE);
smallfish[i].y = rand() % (WIN_HEIGHT - SMALLFISH_SIZE);
smallfish[i].size = SMALLFISH_SIZE;
smallfish[i].speed = rand() % 5 + 1;
smallfish[i].alive = 1;
break;
}
}
}
// 播放背景音乐
void play_bgm() {
PlaySound(TEXT(BGM_FILE), NULL, SND_FILENAME | SND_ASYNC | SND_LOOP);
}
// 播放吃小鱼的声音
void play_eat_sound() {
PlaySound(TEXT(EAT_FILE), NULL, SND_FILENAME | SND_ASYNC);
}
// 保存得分
void save_score(int score, char* name) {
FILE* fp = fopen("score.txt", "a");
if (fp) {
fprintf(fp, "%s %d\n", name, score);
fclose(fp);
}
}
// 显示排行榜
void show_rank() {
cleardevice();
settextstyle(20, 0, "宋体");
outtextxy(10, 10, "排行榜:");
FILE* fp = fopen("score.txt", "r");
if (fp) {
int i = 0;
while (!feof(fp) && i < 10) {
fscanf(fp, "%s %d", rank[i].name, &rank[i].score);
char rank_str[20];
sprintf(rank_str, "%d. %s %d", i + 1, rank[i].name, rank[i].score);
outtextxy(10, 40 + i * 30, rank_str);
i++;
}
fclose(fp);
}
getch();
}
```
注意,以上代码只是一个简单的示例,还有很多细节需要完善,如边界检测、生命值、游戏结束等。同时,也可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐



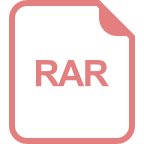
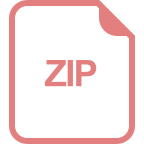
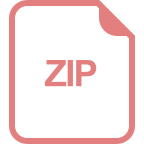









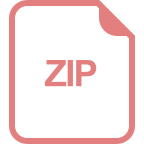