帮我用C语言做一个大鱼吃小鱼游戏
时间: 2023-08-19 17:39:17 浏览: 114
好的,这是一个比较经典的游戏,我可以提供一些基本的思路和代码来帮助您完成这个游戏。
游戏规则:
1. 游戏中有一条大鱼和若干条小鱼。
2. 大鱼可以通过键盘控制上下左右移动,吃掉小鱼可以增加分数。
3. 大鱼碰到边界或者碰到比自己大的鱼游戏结束。
实现思路:
1. 使用C语言的图形库,比如graphics.h来绘制游戏界面。
2. 定义大鱼和小鱼的结构体,包括坐标、大小、速度等属性。
3. 通过键盘监听事件来控制大鱼的移动,检测大鱼和小鱼的碰撞。
4. 绘制分数和游戏结束的提示信息。
代码示例:
```c
#include <graphics.h>
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_FISH_NUM 20
#define WIDTH 600
#define HEIGHT 400
#define FISH_SIZE 30 // 鱼的大小
#define BIG_FISH_SIZE 50 // 大鱼的大小
#define BIG_FISH_SPEED 10 // 大鱼的移动速度
#define SMALL_FISH_SPEED 5 // 小鱼的移动速度
#define SCORE_Y 20 // 分数显示的y坐标
struct Fish {
int x;
int y;
int size;
int speed;
int is_alive; // 是否存活
};
struct Fish big_fish; // 大鱼
struct Fish small_fish[MAX_FISH_NUM]; // 小鱼
int score = 0; // 得分
// 生成小鱼
void generate_small_fish() {
int i;
for (i = 0; i < MAX_FISH_NUM; i++) {
small_fish[i].x = rand() % WIDTH;
small_fish[i].y = rand() % HEIGHT;
small_fish[i].size = FISH_SIZE;
small_fish[i].speed = SMALL_FISH_SPEED;
small_fish[i].is_alive = 1;
}
}
// 初始化游戏
void init_game() {
srand((unsigned int) time(NULL));
big_fish.x = WIDTH / 2;
big_fish.y = HEIGHT / 2;
big_fish.size = BIG_FISH_SIZE;
big_fish.speed = BIG_FISH_SPEED;
big_fish.is_alive = 1;
generate_small_fish();
}
// 绘制大鱼
void draw_big_fish() {
setfillcolor(YELLOW);
fillcircle(big_fish.x, big_fish.y, big_fish.size);
}
// 绘制小鱼
void draw_small_fish() {
int i;
for (i = 0; i < MAX_FISH_NUM; i++) {
if (small_fish[i].is_alive) {
setfillcolor(BLUE);
fillcircle(small_fish[i].x, small_fish[i].y, small_fish[i].size);
}
}
}
// 移动大鱼
void move_big_fish(int x, int y) {
big_fish.x += x * big_fish.speed;
big_fish.y += y * big_fish.speed;
if (big_fish.x < big_fish.size || big_fish.x > WIDTH - big_fish.size ||
big_fish.y < big_fish.size || big_fish.y > HEIGHT - big_fish.size) {
big_fish.is_alive = 0;
}
}
// 移动小鱼
void move_small_fish() {
int i;
for (i = 0; i < MAX_FISH_NUM; i++) {
if (small_fish[i].is_alive) {
int x = rand() % 3 - 1;
int y = rand() % 3 - 1;
small_fish[i].x += x * small_fish[i].speed;
small_fish[i].y += y * small_fish[i].speed;
if (small_fish[i].x < small_fish[i].size || small_fish[i].x > WIDTH - small_fish[i].size ||
small_fish[i].y < small_fish[i].size || small_fish[i].y > HEIGHT - small_fish[i].size) {
small_fish[i].is_alive = 0;
}
}
}
}
// 检测大鱼和小鱼的碰撞
void check_collision() {
int i;
for (i = 0; i < MAX_FISH_NUM; i++) {
if (small_fish[i].is_alive) {
int dx = big_fish.x - small_fish[i].x;
int dy = big_fish.y - small_fish[i].y;
int distance = (int) sqrt(dx * dx + dy * dy);
if (distance < big_fish.size - small_fish[i].size) {
small_fish[i].is_alive = 0;
score++;
} else if (distance < big_fish.size + small_fish[i].size) {
if (big_fish.size > small_fish[i].size) {
small_fish[i].is_alive = 0;
score++;
big_fish.size += 2;
} else {
big_fish.is_alive = 0;
}
}
}
}
}
// 绘制分数
void draw_score() {
char score_str[20];
sprintf(score_str, "Score: %d", score);
outtextxy(10, SCORE_Y, score_str);
}
// 显示游戏结束
void show_game_over() {
setbkcolor(WHITE);
settextcolor(RED);
settextstyle(50, 0, "宋体");
outtextxy(WIDTH / 2 - 150, HEIGHT / 2 - 50, "Game Over!");
}
int main() {
initgraph(WIDTH, HEIGHT);
init_game();
while (1) {
cleardevice();
draw_big_fish();
draw_small_fish();
check_collision();
draw_score();
if (big_fish.is_alive) {
if (kbhit()) {
int c = getch();
switch (c) {
case 'W':
case 'w':
move_big_fish(0, -1);
break;
case 'S':
case 's':
move_big_fish(0, 1);
break;
case 'A':
case 'a':
move_big_fish(-1, 0);
break;
case 'D':
case 'd':
move_big_fish(1, 0);
break;
}
}
move_small_fish();
} else {
show_game_over();
break;
}
delay(50);
}
getch();
closegraph();
return 0;
}
```
上面的代码是一个简单的大鱼吃小鱼游戏的实现,可以根据自己的需求进行修改和完善。
阅读全文
相关推荐
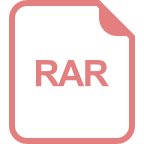
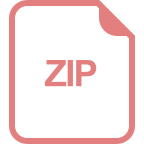




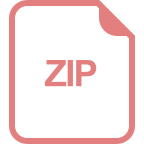
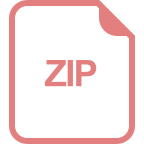







