如何用c语言写大鱼吃小鱼
时间: 2023-05-30 18:03:47 浏览: 257
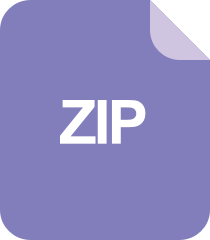
这是一个用java写的大鱼吃小鱼游戏,目的是回顾刚学习完的JavaSE知识,同时增加点乐趣.zip
以下是一个简单的大鱼吃小鱼游戏的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#define BOARD_WIDTH 80
#define BOARD_HEIGHT 20
typedef struct _fish {
int x, y;
int size;
int speed;
} Fish;
typedef struct _fish_node {
Fish fish;
struct _fish_node *next;
} FishNode;
typedef struct _board {
FishNode *fish_list;
char board[BOARD_HEIGHT][BOARD_WIDTH];
} Board;
void init_board(Board *board);
void update_board(Board *board);
void draw_board(Board *board);
void add_fish(Board *board, int x, int y, int size, int speed);
void remove_fish(Board *board, FishNode *node);
void move_fish(Board *board, FishNode *node);
void eat_fish(Board *board, FishNode *fish, FishNode *food);
int random_int(int min, int max);
int main()
{
srand((unsigned)time(NULL));
Board board;
init_board(&board);
add_fish(&board, BOARD_WIDTH / 2, BOARD_HEIGHT / 2, 5, 1);
while (1) {
if (_kbhit()) {
char key = _getch();
if (key == 'q' || key == 'Q')
break;
}
update_board(&board);
draw_board(&board);
Sleep(100);
}
return 0;
}
void init_board(Board *board)
{
board->fish_list = NULL;
for (int i = 0; i < BOARD_HEIGHT; i++) {
for (int j = 0; j < BOARD_WIDTH; j++) {
if (i == 0 || i == BOARD_HEIGHT - 1)
board->board[i][j] = '-';
else if (j == 0 || j == BOARD_WIDTH - 1)
board->board[i][j] = '|';
else
board->board[i][j] = ' ';
}
}
}
void update_board(Board *board)
{
FishNode *fish = board->fish_list;
while (fish != NULL) {
move_fish(board, fish);
FishNode *food = board->fish_list;
while (food != NULL) {
if (food != fish && fish->fish.size > food->fish.size) {
int dx = fish->fish.x - food->fish.x;
int dy = fish->fish.y - food->fish.y;
if (dx * dx + dy * dy < fish->fish.size * fish->fish.size) {
eat_fish(board, fish, food);
break;
}
}
food = food->next;
}
fish = fish->next;
}
}
void draw_board(Board *board)
{
system("cls");
for (int i = 0; i < BOARD_HEIGHT; i++) {
for (int j = 0; j < BOARD_WIDTH; j++) {
putchar(board->board[i][j]);
}
putchar('\n');
}
}
void add_fish(Board *board, int x, int y, int size, int speed)
{
FishNode *node = (FishNode*)malloc(sizeof(FishNode));
node->fish.x = x;
node->fish.y = y;
node->fish.size = size;
node->fish.speed = speed;
node->next = board->fish_list;
board->fish_list = node;
board->board[y][x] = 'O';
}
void remove_fish(Board *board, FishNode *node)
{
FishNode *prev = NULL;
FishNode *curr = board->fish_list;
while (curr != NULL) {
if (curr == node) {
if (prev != NULL)
prev->next = curr->next;
else
board->fish_list = curr->next;
free(curr);
break;
}
prev = curr;
curr = curr->next;
}
}
void move_fish(Board *board, FishNode *node)
{
int dx = random_int(-1, 1);
int dy = random_int(-1, 1);
int x = node->fish.x + dx * node->fish.speed;
int y = node->fish.y + dy * node->fish.speed;
if (x >= 1 && x < BOARD_WIDTH - 1 && y >= 1 && y < BOARD_HEIGHT - 1) {
board->board[node->fish.y][node->fish.x] = ' ';
node->fish.x = x;
node->fish.y = y;
board->board[y][x] = 'O';
}
}
void eat_fish(Board *board, FishNode *fish, FishNode *food)
{
fish->fish.size += food->fish.size;
fish->fish.speed += food->fish.size / 5;
remove_fish(board, food);
}
int random_int(int min, int max)
{
return rand() % (max - min + 1) + min;
}
```
这个程序使用了一个简单的结构体来表示鱼,包括鱼的位置、大小和速度。鱼在游戏板上移动,如果两条鱼的距离小于它们的大小之和,那么它们就会碰撞,大鱼会吃掉小鱼。程序使用一个链表来存储所有的鱼,每次更新游戏板时遍历链表,移动每条鱼并检查是否有碰撞发生。如果发生了碰撞,那么程序就会让大鱼吃掉小鱼。程序使用了标准库中的一些函数,包括`stdlib.h`中的`malloc()`和`free()`函数来分配和释放内存,`conio.h`中的`_kbhit()`和`_getch()`函数来读取按键输入,以及`time.h`中的`srand()`函数来初始化随机数生成器。
阅读全文
相关推荐


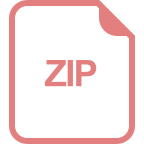







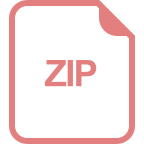
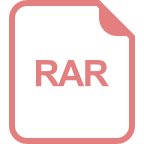
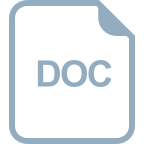


