大鱼吃小鱼C语言程序数据结构顺序撰写
时间: 2023-07-07 07:42:17 浏览: 350
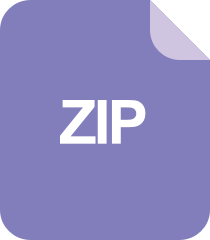
【c语言程序设计课设 大鱼吃小鱼 完整源程序+设计报告】
大鱼吃小鱼是一个经典的游戏,它的实现可以采用链表数据结构。下面是一个简单的C语言程序实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义鱼的结构体
struct Fish {
int size; // 鱼的大小
struct Fish *next; // 指向下一个鱼的指针
};
// 创建一条新鱼
struct Fish *createFish(int size) {
struct Fish *fish = (struct Fish*)malloc(sizeof(struct Fish));
fish->size = size;
fish->next = NULL;
return fish;
}
// 吃掉一条鱼
void eatFish(struct Fish *fish, struct Fish **pond) {
struct Fish *prev = NULL;
struct Fish *current = *pond;
// 找到比自己小的鱼
while (current != NULL && current->size > fish->size) {
prev = current;
current = current->next;
}
// 如果找到了比自己小的鱼,吃掉它
if (current != NULL && current->size <= fish->size) {
if (prev != NULL) {
prev->next = current->next;
} else {
*pond = current->next;
}
free(current);
}
}
// 打印池塘中的鱼
void printFish(struct Fish *pond) {
while (pond != NULL) {
printf("%d ", pond->size);
pond = pond->next;
}
printf("\n");
}
int main() {
// 初始化池塘中的鱼
struct Fish *fish1 = createFish(10);
struct Fish *fish2 = createFish(5);
struct Fish *fish3 = createFish(3);
struct Fish *fish4 = createFish(8);
struct Fish *pond = fish1;
fish1->next = fish2;
fish2->next = fish3;
fish3->next = fish4;
// 打印初始状态
printf("初始状态:\n");
printFish(pond);
// 大鱼吃小鱼
eatFish(fish1, &pond);
// 打印最终状态
printf("最终状态:\n");
printFish(pond);
return 0;
}
```
在这个程序中,我们首先定义了一个鱼的结构体,包含鱼的大小和指向下一个鱼的指针。然后,我们实现了三个函数:`createFish`用于创建一条新鱼,`eatFish`用于让大鱼吃小鱼,`printFish`用于打印池塘中的鱼。
在`main`函数中,我们首先初始化池塘中的鱼,然后打印初始状态。接着,我们让大鱼(即第一条鱼)吃小鱼,最后打印最终状态。
阅读全文
相关推荐
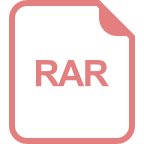








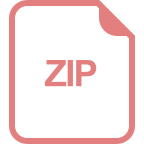
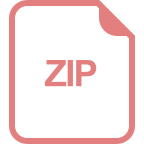





