给我在网络上搜索一个可以运行的大鱼吃小鱼C语言程序
时间: 2024-05-07 11:22:45 浏览: 77
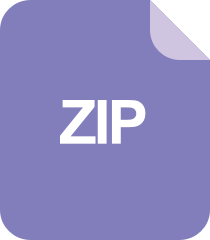
大鱼吃小鱼游戏程序设计

以下是一个可以在网络上找到的大鱼吃小鱼C语言程序的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#define WIDTH 50
#define HEIGHT 20
#define FOOD_NUM 20
typedef struct Node {
int x;
int y;
struct Node* next;
}Node;
enum Direction {
UP,
DOWN,
LEFT,
RIGHT
};
int Score = 0;
int FoodCount = 0;
int Map[HEIGHT][WIDTH] = {0};
Node* Head = NULL;
Node* Tail = NULL;
Node* Foods[FOOD_NUM] = {NULL};
void DrawMap() {
system("cls");
printf("Score: %d\n", Score);
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (Map[i][j] == 0) {
printf(" ");
} else if (Map[i][j] == 1) {
printf("*");
} else if (Map[i][j] == 2) {
printf("#");
} else if (Map[i][j] == 3) {
printf("@");
}
}
printf("\n");
}
}
void InitSnake() {
Head = (Node*) malloc(sizeof(Node));
Tail = Head;
Head->x = WIDTH / 2;
Head->y = HEIGHT / 2;
Head->next = NULL;
Map[Head->y][Head->x] = 1;
}
void AddTail(int x, int y) {
Node* node = (Node*) malloc(sizeof(Node));
node->x = x;
node->y = y;
node->next = NULL;
Tail->next = node;
Tail = node;
Map[y][x] = 1;
}
void RemoveTail() {
Node* node = Head;
while (node->next != Tail) {
node = node->next;
}
Map[Tail->y][Tail->x] = 0;
free(Tail);
Tail = node;
Tail->next = NULL;
}
void InitFood() {
srand((unsigned)time(NULL));
for (int i = 0; i < FOOD_NUM; i++) {
Foods[i] = (Node*) malloc(sizeof(Node));
Foods[i]->x = rand() % WIDTH;
Foods[i]->y = rand() % HEIGHT;
Foods[i]->next = NULL;
Map[Foods[i]->y][Foods[i]->x] = 2;
FoodCount++;
}
}
int EatFood(int x, int y) {
int score = 0;
for (int i = 0; i < FOOD_NUM; i++) {
if (Foods[i] && Foods[i]->x == x && Foods[i]->y == y) {
score++;
Map[y][x] = 0;
free(Foods[i]);
Foods[i] = NULL;
FoodCount--;
}
}
return score;
}
int MoveSnake(enum Direction dir) {
int new_x = Head->x;
int new_y = Head->y;
switch (dir) {
case UP:
new_y--;
break;
case DOWN:
new_y++;
break;
case LEFT:
new_x--;
break;
case RIGHT:
new_x++;
break;
}
if (new_x < 0 || new_x >= WIDTH || new_y < 0 || new_y >= HEIGHT) {
return 0;
}
if (Map[new_y][new_x] == 1) {
return 0;
}
int score = EatFood(new_x, new_y);
AddTail(new_x, new_y);
if (score == 0) {
RemoveTail();
} else {
Score += score;
}
Head = Tail;
Tail = Tail->next;
Map[new_y][new_x] = 1;
return 1;
}
int main() {
InitSnake();
InitFood();
DrawMap();
while (1) {
if (_kbhit()) {
enum Direction dir = UP;
char c = _getch();
switch (c) {
case 'w':
dir = UP;
break;
case 's':
dir = DOWN;
break;
case 'a':
dir = LEFT;
break;
case 'd':
dir = RIGHT;
break;
case 'q':
return 0;
}
if (!MoveSnake(dir)) {
printf("Game Over!\n");
return 0;
}
DrawMap();
}
}
return 0;
}
```
注意:这是一个控制台程序,需要在控制台中运行。
阅读全文
相关推荐
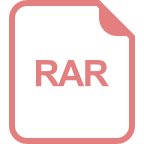








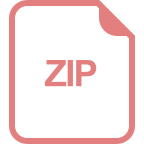
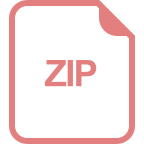
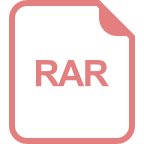



