已知一个固定容量的背包c=8和3个物品,每个物品有其重量和价值,重量分别为 (2,了,1),价值分别为(1,9,3)。如果每个 物品只能拿1个或不拿,请利用优先队列式分 支限界法求不超过背包容量的前提下可获得 的最大价值,用c++设计带注释剪枝函数
时间: 2024-05-01 21:22:37 浏览: 104
优先队列式分支限界法是一种常用的求解01背包问题的算法。它的核心思想是通过优先队列来维护当前状态的子节点,每次取出队首元素进行拓展,直到队列为空或者找到最优解。在拓展过程中,通过剪枝函数对可能不符合要求的状态进行剪枝,从而减少搜索空间,提高算法效率。
下面给出一个示例代码,具体实现见注释:
```c++
#include <iostream>
#include <queue>
using namespace std;
// 物品结构体
struct Item {
int weight; // 重量
int value; // 价值
double ratio; // 价值重量比,用于排序
};
// 剪枝函数,判断当前状态是否符合要求
bool pruning(int capacity, int current_weight, int current_value, priority_queue<Item> pq) {
// 如果当前重量已经超过了背包容量,则剪枝
if (current_weight > capacity) {
return true;
}
// 计算剩余物品的最大价值上限
double max_value = current_value;
int remaining_capacity = capacity - current_weight;
while (!pq.empty() && remaining_capacity >= pq.top().weight) {
max_value += pq.top().value;
remaining_capacity -= pq.top().weight;
pq.pop();
}
// 如果剩余物品的最大价值上限仍然小于当前价值,则剪枝
if (max_value < current_value) {
return true;
}
return false;
}
// 优先队列式分支限界法
int knapsack(int capacity, int n, int* weight, int* value) {
// 将物品按照价值重量比从大到小排序,便于优先队列的弹出
priority_queue<Item> pq;
for (int i = 0; i < n; i++) {
Item item = {weight[i], value[i], (double)value[i] / weight[i]};
pq.push(item);
}
// 初始化队首元素
Item current_item = pq.top();
pq.pop();
// 初始化状态
int current_weight = 0;
int current_value = 0;
int max_value = 0;
// 优先队列式分支限界法主循环
while (true) {
// 判断当前状态是否符合要求
if (!pruning(capacity, current_weight, current_value, pq)) {
// 如果符合要求,则更新最大价值和当前状态
if (current_value > max_value) {
max_value = current_value;
}
// 如果队列为空,则搜索结束
if (pq.empty()) {
break;
}
// 取出队首元素进行拓展
Item next_item = pq.top();
pq.pop();
// 分别考虑取和不取两种情况
if (current_weight + next_item.weight <= capacity) {
pq.push(current_item);
current_item = next_item;
current_weight += current_item.weight;
current_value += current_item.value;
} else {
double remaining_value = (double)next_item.value / next_item.weight * (capacity - current_weight);
pq.push({capacity - current_weight, (int)remaining_value, remaining_value});
}
} else {
// 如果不符合要求,则直接弹出队首元素
if (pq.empty()) {
break;
}
current_item = pq.top();
pq.pop();
}
}
return max_value;
}
int main() {
int capacity = 8;
int n = 3;
int weight[] = {2, 5, 1};
int value[] = {1, 9, 3};
int max_value = knapsack(capacity, n, weight, value);
cout << "Max value: " << max_value << endl;
return 0;
}
```
本代码中的剪枝函数采用了两种剪枝策略。首先,对于当前状态,如果其已经超过了背包容量,则直接剪枝。其次,计算剩余物品的最大价值上限,如果该上限仍然小于当前价值,则也可以剪枝。在实际应用中,还可以采用其他剪枝策略,如动态规划剪枝、可行性剪枝等,以进一步提高算法效率。
阅读全文
相关推荐
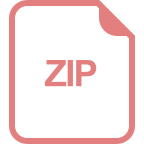
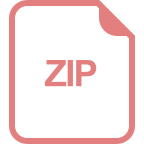
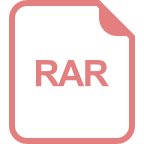


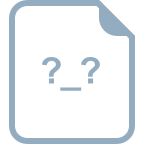
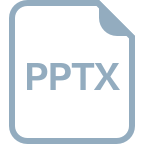
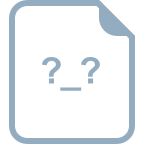
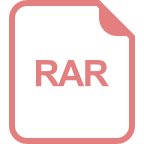
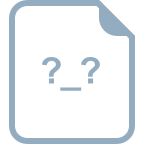
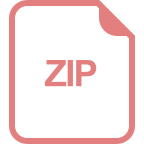
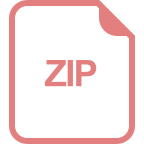
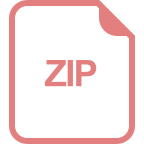
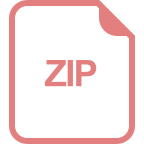
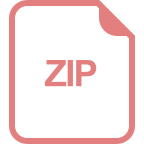
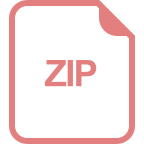
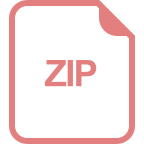