用Python实现五子棋游戏
时间: 2023-11-05 20:03:52 浏览: 99
以下是一种可能的实现方式:
```python
import pygame
# 设置游戏界面尺寸
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 640
# 设置棋盘尺寸和格子大小
BOARD_SIZE = 15
GRID_SIZE = SCREEN_WIDTH // BOARD_SIZE
# 定义棋子颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
# 初始化 pygame
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("五子棋")
# 画棋盘
def draw_board():
for i in range(BOARD_SIZE):
pygame.draw.line(screen, BLACK, (GRID_SIZE // 2, GRID_SIZE // 2 + i * GRID_SIZE),
(SCREEN_WIDTH - GRID_SIZE // 2, GRID_SIZE // 2 + i * GRID_SIZE))
pygame.draw.line(screen, BLACK, (GRID_SIZE // 2 + i * GRID_SIZE, GRID_SIZE // 2),
(GRID_SIZE // 2 + i * GRID_SIZE, SCREEN_HEIGHT - GRID_SIZE // 2))
# 下棋子
def place_piece(grid_x, grid_y, color):
x = GRID_SIZE // 2 + grid_x * GRID_SIZE
y = GRID_SIZE // 2 + grid_y * GRID_SIZE
pygame.draw.circle(screen, color, (x, y), GRID_SIZE // 2 - 2)
# 判断胜利条件
def check_win(board, row, col):
directions = [(0, 1), (1, 0), (1, 1), (1, -1)]
for d in directions:
count = 1
for i in range(1, 5):
r = row + i * d[0]
c = col + i * d[1]
if r < 0 or r >= BOARD_SIZE or c < 0 or c >= BOARD_SIZE or board[r][c] != board[row][col]:
break
count += 1
for i in range(1, 5):
r = row - i * d[0]
c = col - i * d[1]
if r < 0 or r >= BOARD_SIZE or c < 0 or c >= BOARD_SIZE or board[r][c] != board[row][col]:
break
count += 1
if count >= 5:
return True
return False
# 初始化游戏状态
board = [[0] * BOARD_SIZE for _ in range(BOARD_SIZE)]
current_player = 1
game_over = False
winner = None
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.MOUSEBUTTONDOWN and not game_over:
x, y = event.pos
grid_x, grid_y = x // GRID_SIZE, y // GRID_SIZE
if board[grid_y][grid_x] == 0:
place_piece(grid_x, grid_y, BLACK if current_player == 1 else WHITE)
board[grid_y][grid_x] = current_player
if check_win(board, grid_y, grid_x):
game_over = True
winner = current_player
else:
current_player = 2 if current_player == 1 else 1
# 绘制游戏界面
screen.fill(WHITE)
draw_board()
for y in range(BOARD_SIZE):
for x in range(BOARD_SIZE):
if board[y][x] == 1:
place_piece(x, y, BLACK)
elif board[y][x] == 2:
place_piece(x, y, WHITE)
if game_over:
font = pygame.font.SysFont(None, 48)
text = font.render("Player " + str(winner) + " wins!", True, BLACK)
screen.blit(text, (SCREEN_WIDTH // 2 - text.get_width() // 2, SCREEN_HEIGHT // 2 - text.get_height() // 2))
pygame.display.update()
```
这个程序使用了 Pygame 库来实现游戏界面和交互。程序初始化了游戏状态,然后在游戏循环中处理游戏事件,绘制游戏界面,并检查胜利条件。游戏界面由一个棋盘和棋子组成,棋盘是由若干个格子组成的,每个格子大小为 `GRID_SIZE`,棋子半径为 `GRID_SIZE // 2 - 2`。棋盘的大小和格子大小都可以通过调整常量来修改。棋盘上的每个格子被保存在一个二维数组 `board` 中,初始值为 0,表示该格子为空。玩家下棋时,程序会检查该位置是否为空,如果是,则绘制该玩家的棋子,并将该位置的值设为该玩家的编号(1 或 2)。下棋后,程序会检查胜利条件,如果满足条件,则游戏结束,记录胜利者。游戏结束后,程序会在界面上显示胜利者的信息。
阅读全文
相关推荐
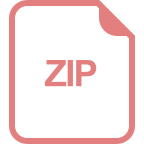
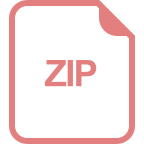
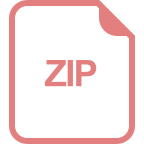
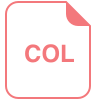

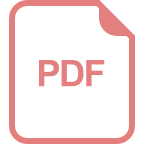
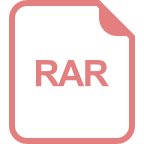
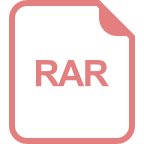
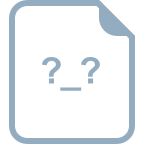
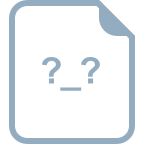
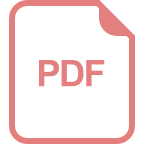
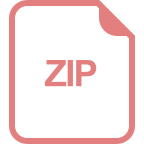
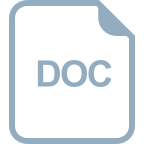
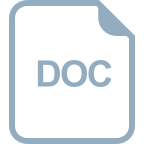
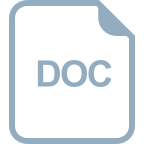
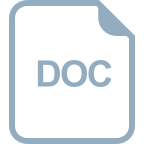
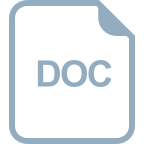

