stream抽取map
时间: 2023-08-05 12:06:18 浏览: 60
list通过stream转换成map的方法如下:
1. 首先,使用stream()方法将list转换为一个流。
2. 然后,使用collect()方法将流转换为一个map。在collect()方法中,使用Collectors.toMap()方法将流中的元素转换为map中的键值对。
例如,假设有一个List<Person>,其中Person类有两个属性:name和age。现在要将这个List转换为一个Map<String, Integer>,其中键是Person的name属性,值是Person的age属性。可以使用以下代码:
Map<String, Integer> map = list.stream()
.collect(Collectors.toMap(Person::getName, Person::getAge));
其中,Person::getName和Person::getAge是方法引用,用于获取Person对象的name和age属性。这样,就可以将List转换为Map了。
相关问题
list嵌套map 使用stream的map方法切割数据
假设你有一个嵌套的List<Map>数据结构,你想使用Stream的map方法来切割数据。下面是一个示例代码:
```java
List<Map<String, Object>> data = new ArrayList<>();
Map<String, Object> map1 = new HashMap<>();
map1.put("name", "Alice");
map1.put("age", 25);
Map<String, Object> map2 = new HashMap<>();
map2.put("name", "Bob");
map2.put("age", 30);
data.add(map1);
data.add(map2);
List<String> names = data.stream()
.map(m -> m.get("name").toString())
.collect(Collectors.toList());
List<Integer> ages = data.stream()
.map(m -> Integer.parseInt(m.get("age").toString()))
.collect(Collectors.toList());
```
在上面的示例中,我们首先创建了一个嵌套的List<Map>数据结构,其中每个Map表示一个人的姓名和年龄。然后,我们使用Stream的map方法来抽取每个Map中的姓名和年龄字段,并分别将它们收集到两个不同的List中。
注意,在使用map方法时,我们需要使用lambda表达式来指定映射函数,以便从每个Map中提取所需的数据。在本例中,我们使用m -> m.get("name").toString()来提取姓名,使用m -> Integer.parseInt(m.get("age").toString())来提取年龄。最后,我们使用Collectors.toList()来将结果收集到List中。
希望这可以帮助你解决问题!
不用遍历的方式在对象集合list中抽取属性集合转化为map或list
可以使用Java 8中的Stream API来实现,具体方法如下:
1. 如果要抽取对象的某一个属性,可以使用map方法将对象转换成属性,然后使用collect方法将属性收集成一个集合。例如,假设有一个Student类,其中包含name和age两个属性,需要将所有学生的姓名抽取出来转化成一个List,可以使用以下代码:
```
List<Student> students = ...;
List<String> names = students.stream()
.map(Student::getName)
.collect(Collectors.toList());
```
2. 如果要抽取对象的多个属性,可以将多个属性封装成一个对象,然后使用map方法将对象转换成属性集合,最后使用collect方法将属性集合收集成一个集合。例如,假设有一个Student类,其中包含name和age两个属性,需要将所有学生的姓名和年龄抽取出来转化成一个Map,可以使用以下代码:
```
List<Student> students = ...;
Map<String, Integer> nameAgeMap = students.stream()
.map(s -> new AbstractMap.SimpleEntry<>(s.getName(), s.getAge()))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
```
这里使用了AbstractMap.SimpleEntry类来封装属性,然后使用toMap方法将属性集合转化为Map。如果属性集合中存在重复的属性,可以使用toMap方法的第三个参数来指定如何处理重复的属性。
相关推荐
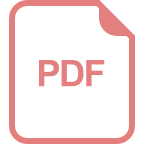
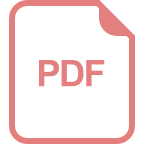
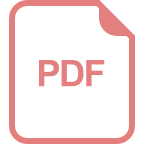









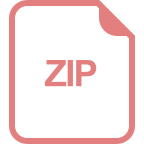